Have you ever been looking for a easy answer on the best way to monitor all ETH switch transactions? In that case, you’ve come to the best place! Due to the Moralis Streams API, you may simply monitor ETH transactions, and you are able to do so both through Moralis’ user-friendly UI or SDK. What’s extra, even once you select the SDK path, you solely have to arrange just a few strains of code to get the job completed! For instance, these are the snippets of code to make use of when working with JavaScript:
const newStream = await Moralis.Streams.add(choices)
const {id}=newStream.toJSON();
const tackle=”wallet_address_you_want_to_track”
await Moralis.Streams.addAddress({tackle,id})
So, in case you’ve been questioning the best way to monitor all ETH switch transactions, you may see from the above instance that Moralis is the best choice to take action and fetch Web3 real-time knowledge! Now, in case you labored with Moralis Web3 APIs earlier than, you already know the best way to implement the above strains of code. Nevertheless, if that is your first rodeo with this final Web3 API supplier, ensure that to look at the video on the prime or dive into the next sections.
Overview
Shifting ahead, we’ll dive straight into an instance of the best way to monitor all ETH switch transactions by specializing in crypto pockets monitoring. We are going to strategy this instance in two methods. First, you’ll see the best way to go about it utilizing Moralis’ admin UI. Second, we’ll full the identical feat through the use of the JS SDK.
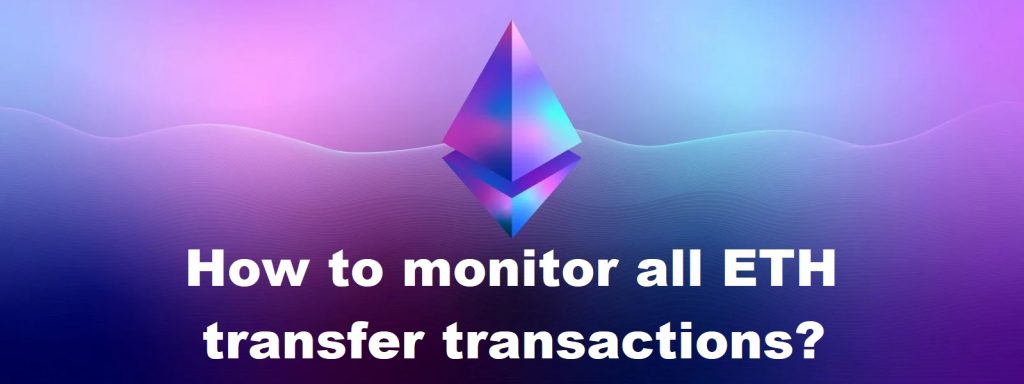
By taking up this instance, you’ll learn to get going with Moralis’ Streams API and the best way to implement the above-outlined strains of code. In any case, you could find a whole code walkthrough under.
Nevertheless, under at present’s tutorial, you could find further sections explaining extra about the best way to monitor crypto transactions. Apart from specializing in explicit wallets, you too can concentrate on a specific cryptocurrency (e.g., ETH). Primarily, you may monitor any fungible and non-fungible token sensible contract and obtain notifications each time the token in query is transferred.
Tutorial: The way to Monitor All ETH Switch Transactions with the Moralis Streams API
Whether or not you propose on utilizing Moralis’ admin UI or SDK, you want an energetic Moralis account. Thus, earlier than shifting ahead, ensure that to create your free Moralis account. Merely click on on the hyperlink within the earlier sentence or hit the “Begin for Free” button on the far right-hand aspect of the Moralis web site prime menu:

Additionally, for the sake of this tutorial, we’ll concentrate on the Polygon testnet. Nevertheless, the rules are the identical in case you concentrate on the Ethereum mainnet or some other EVM-compatible chain. With that stated, in case you want to comply with our lead, ensure that to get some “take a look at” MATIC through the use of the Polygon Mumbai faucet that awaits you on the Moralis Pure Taps web page.
Observe: Be at liberty to concentrate on some other chain. Nevertheless, we strongly suggest that you just begin by focusing on testnets.
ETH Switch Monitoring with Moralis Admin UI – Getting Began
Together with your Moralis account up and working, you’ll have the ability to entry your admin space. Be at liberty to start out with the “Get Began” tutorial that awaits you on the within. Or, merely hit the “Create a challenge” button and identify your challenge:
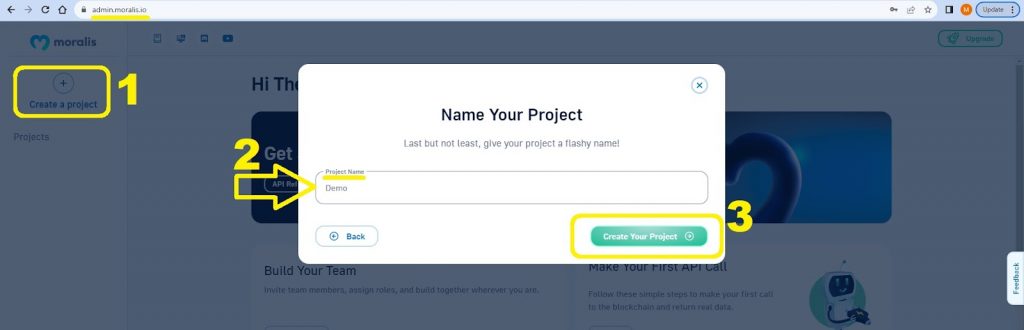
After creating your challenge, you may concentrate on the “the best way to monitor all ETH switch transactions” problem. To take action, choose the Streams choice from the aspect menu after which click on on one of many two “Create a brand new Stream” buttons:
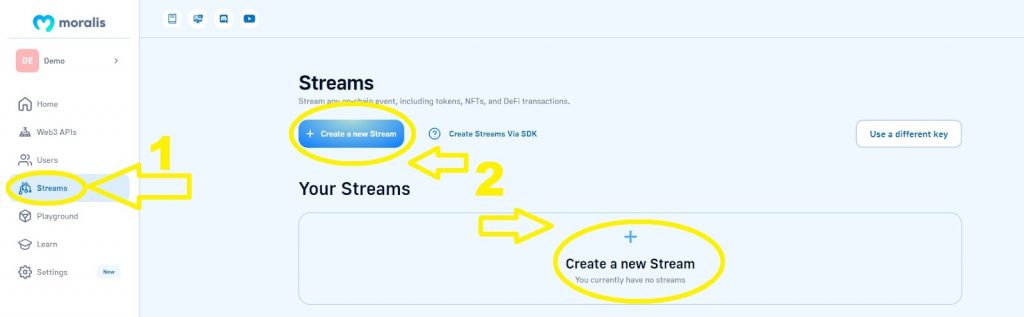
Subsequent, it’s essential to enter an Ethereum tackle you want to monitor. This generally is a pockets tackle or a token sensible contract tackle. For the sake of this tutorial, we’ll concentrate on pockets monitoring. So, be happy to make use of any pockets tackle that you really want.
Nevertheless, for academic and testing functions, utilizing a pockets tackle you management is the best choice. So, in case you use MetaMask, merely copy your tackle.
To proceed, merely paste the tackle into the “Add EVM/Aptos Handle to Stream” entry subject:
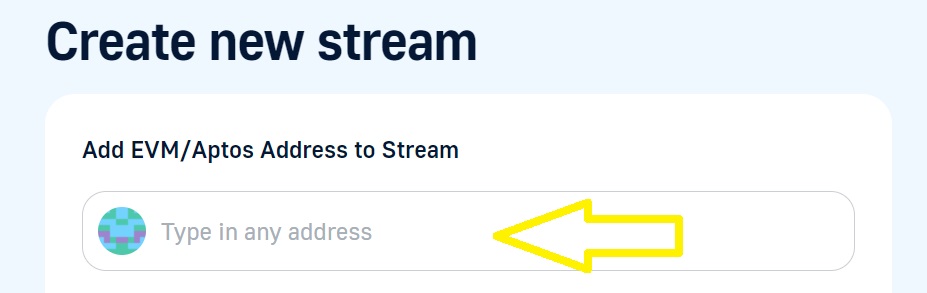
As quickly as you paste an tackle into the designated fields, the UI will routinely current you with stream configuration choices.
Stream Setup
As indicated within the picture under, you may toggle between “Demo” and “Prod”, amongst different choices. When you’re prepared to make use of this characteristic for manufacturing functions, you’ll wish to change to “Prod”. Nevertheless, for the sake of this tutorial, stick with “Demo”:
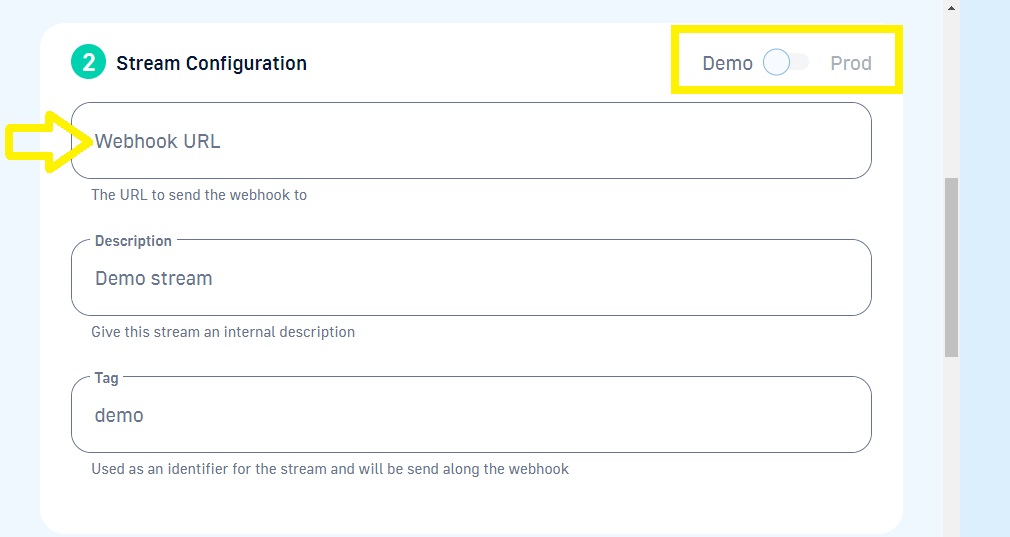
Trying on the above screenshot, you additionally see the “Webhook URL”, “Description”, and “Tag” entry fields. For demonstration functions, you don’t want to offer a webhook URL. As for the outline and tag fields, you may go along with the default choices.
Scrolling additional down, you’ll see the “Choose Networks” part. That is the place you may toggle the buttons subsequent to all of the networks you want to concentrate on. Nevertheless, as identified beforehand, for the sake of this tutorial, we’ll be specializing in Polygon’s testnet (Mumbai):

After deciding on the community(s) you want to concentrate on, you may transfer on to the fourth step of the stream setup course of. Once more, you might have a number of choices:
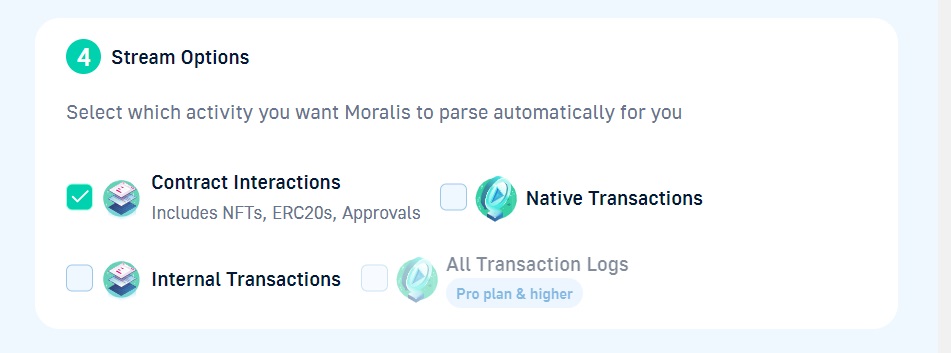
As you may see within the picture above, we determined to concentrate on contract interactions as a result of we’ll do an instance ERC-20 switch. Nevertheless, in case you have been to sort out the “the best way to monitor all ETH switch transactions” activity head-on, you’d want to pick Ethereum in step three and tick the “Native Transactions” field in step 4.
Scrolling additional down your stream setup, you’ll additionally see the “Superior Choices” and “Triggers” sections. The previous lets you filter your monitoring agenda additional through the use of the sensible contract’s ABI to focus on particular sensible contract capabilities.
As for the Moralis triggers, you get to run read-only sensible contract capabilities. By doing so, you get outcomes from these capabilities as a part of your Web3 webhooks.
View the Outcomes – Instance Transaction
With none particular tweaks, you may already see the facility of your new stream. Right here’s our instance transaction of Chainlink (LINK) tokens on the Mumbai testnet:
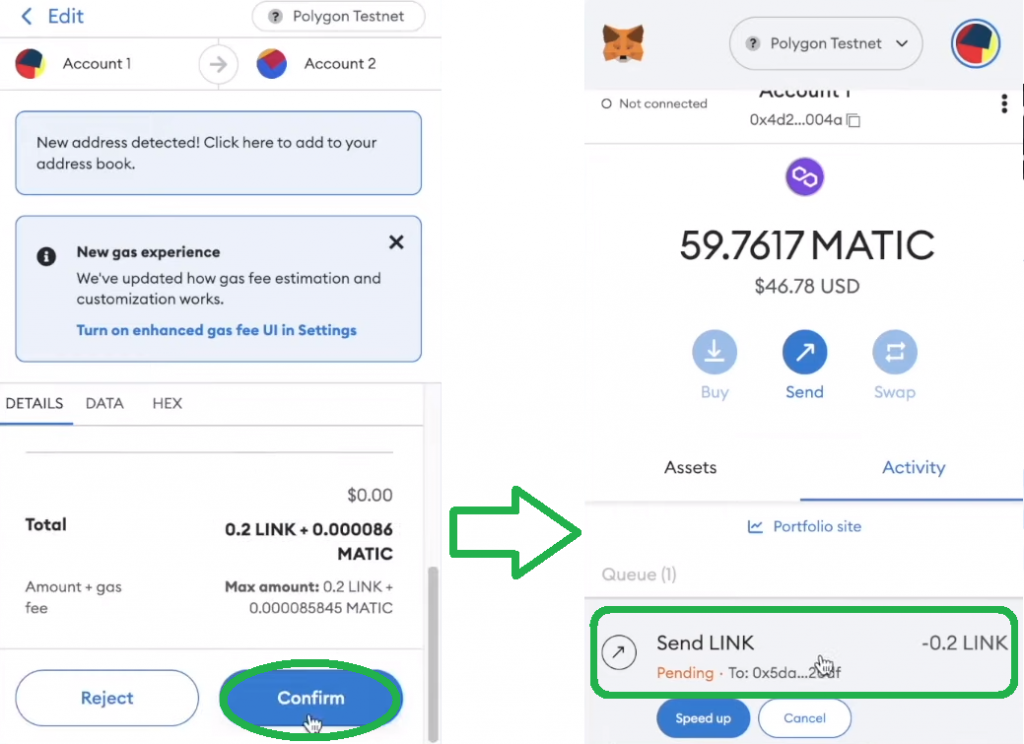
Our demo stream detects the above instance transaction instantaneously:
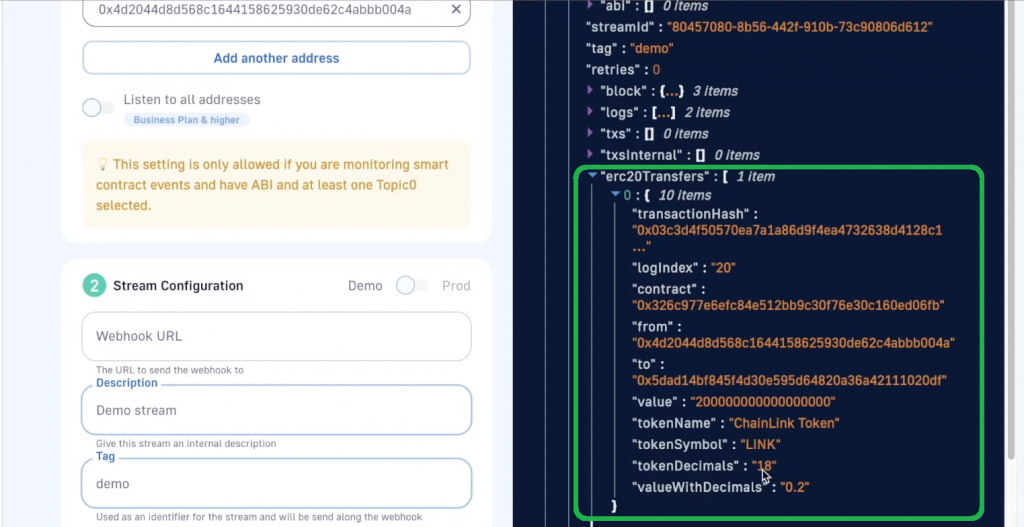
To expertise the facility of this superb device, we encourage you to run your individual instance transaction. Then, you’ll get to see all of the related on-chain knowledge that this kind of monitoring presents (see the above screenshot), together with the transaction hash, the sensible contract concerned within the occasion, the “from” and “to” addresses, the transferred worth, and extra.
Primarily, setting a Moralis stream offers you a robust detector to identify on-chain occasions. And, when any of the occasions happen, you not solely detect them, however you additionally get the associated particulars. As such, you may simply make the most of these particulars to create highly effective bots, auto-messaging dapps, and rather more.
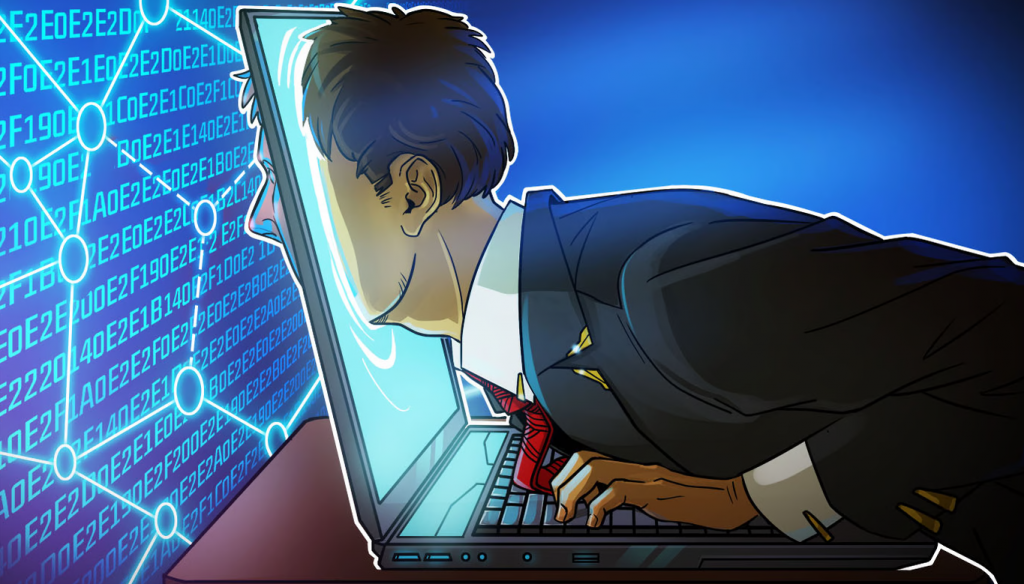
The way to Monitor All ETH Switch Transactions with Moralis’ JS SDK
Though establishing on-chain monitoring through the Moralis admin space is the best path, when constructing extra superior dapps, you would possibly desire to set issues up through Moralis’ SDK.
Observe: If you would like detailed directions on the best way to use Streams through the SDK, hit the related button on the “Stream” web page of your Moralis admin space:
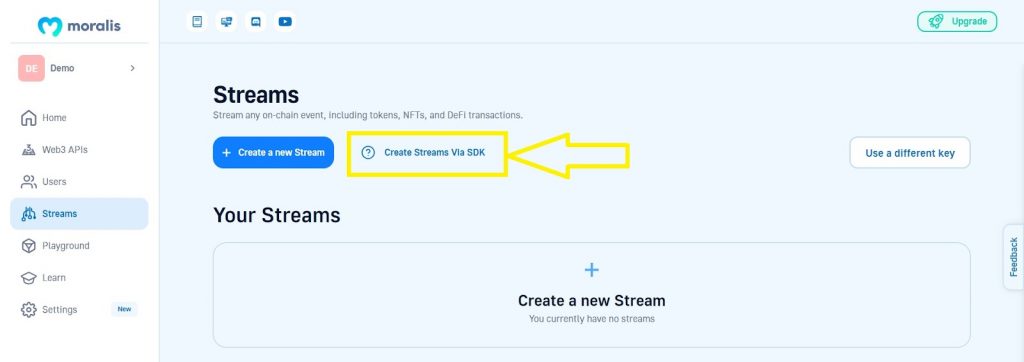
So, you could be questioning the best way to monitor all ETH switch transactions programmatically. First, it’s essential to resolve which programming language/framework you want to use. You could have many choices, although JavaScript or Python mixed with NodeJS tends to be the preferred.
Nevertheless, earlier than you may concentrate on creating your stream through the SDK, it is advisable put together a server. The latter will obtain a webhook. Fortuitously, you may create a easy NodeJS Categorical app, which can offer you a “webhook” endpoint.
Create a Native Server
So, to that finish, you need to use the next strains of code – merely copy-paste them into your “index.js” file:
const categorical = require(“categorical”);
const app = categorical();
const port = 3000;
app.use(categorical.json());
app.publish(“/webhook”, async (req, res) => {
const {physique} = req;
attempt {
console.log(physique);
} catch (e) {
console.log(e);
return res.standing(400).json();
}
return res.standing(200).json();
});
app.hear(port, () => {
console.log(`Listening to streams`);
});
Observe: If you happen to’ve by no means created NodeJS functions and arrange Categorical servers, dive into our “Quickstart NodeJS” information.
With the above script in place, merely enter and run the npm run begin command utilizing your terminal.
In response to the above command, your terminal ought to return the “Listening to streams” message:
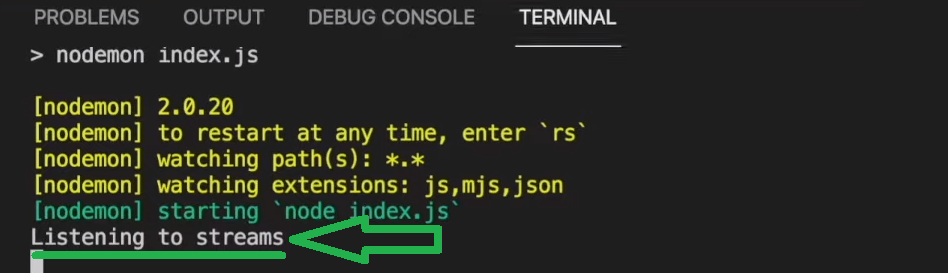
Create a Forwarding Tunnel
Subsequent, open a brand new terminal and run the ngrok http 3000 command. This can create a tunnel to port 3000. In response to this command, you’ll get a URL tackle to make use of as a webhook URL in your stream.

Together with your native server and tunnel prepared, you need to use the strains of code outlined within the upcoming part to watch numerous on-chain actions. And, after all, the “the best way to monitor all ETH switch transactions” feat can also be one of many choices you may concentrate on.
Crypto Switch Monitoring – Code Walkthrough
Begin by initializing one other NodeJS app. Subsequent, create a brand new “index.js” file, which must require Moralis and dotenv:
const Moralis = require(“moralis”).default;
const { EvmChain } = require(“@moralisweb3/common-evm-utils”);
require(“dotenv”).config();
As well as, it is advisable create a “.env” file. Inside that file, retailer your Moralis Web3 API key below the MORALIS_KEY variable. To acquire the worth of that variable, entry your Moralis admin space, go to “Settings”, and duplicate the default API key or create a brand new one:

Now that you’ve your API key, you may initialize Moralis. To take action, add the next strains of code under require (“dotenv”).config();:
Moralis.begin({
apiKey: course of.env.MORALIS_KEY,
});
Then, it’s time to concentrate on the stream’s choices with the next strains of code:
async perform streams(){
const choices = {
chains: [EvmChain.MUMBAI],
tag: “transfers”,
description: “Take heed to Transfers”,
includeContractLogs: false,
includeNativeTxs: true,
webhookUrl: “your webhook url”
}
Trying on the above code snippet, you may see that it defines a sequence to concentrate on, an outline, a tag, and a webhook URL. These are all the identical choices we lined within the above part, as we used Moralis’ admin UI.
We are going to concentrate on listening to native transactions on the Mumbai testnet. So, we set includeContractLogs to false in an effort to not hearken to ERC-20 transactions. Moreover, we concentrate on native foreign money transfers by setting includeNativeTxs to true. Relating to the Mumbai community, the native foreign money is “take a look at” MATIC.
To make use of the above strains of code in your “index.js” script, you simply want to interchange your webhook url with the beforehand generated ngrok URL:

As indicated within the above picture, ensure that so as to add /webhook on the finish of your ngrok URL.
Create a New Stream
By protecting all of the above-outlined steps, you’re lastly able to implement the strains of code showcased within the introduction of this text.
As such, concentrate on the above-defined async perform streams and add the next snippets of code under the webhookUrl line:
const newStream = await Moralis.Streams.add(choices)
const {id} = newStream.toJSON();
const tackle = “wallet_address_you_want_to_track”;
await Moralis.Streams.addAddress({tackle, id})
console.log(“Fin”)
}
streams()
The Moralis.Streams.add(choices) technique makes use of the above-defined stream choice to create a brand new stream. Subsequent, the code makes use of const {id} to fetch the brand new stream’s ID. Plus, it defines a pockets tackle to watch. That is the place it is advisable substitute wallet_address_you_want_to_track with an precise tackle.
The Moralis.Streams.addAddress technique makes use of your stream’s ID to assign the pockets tackle to it. Lastly, the code logs Fin as soon as it completes all of the earlier steps.
To run your script, enter node index.js. If you happen to did the whole lot in accordance with the above directions, you need to see Fin in your terminal:
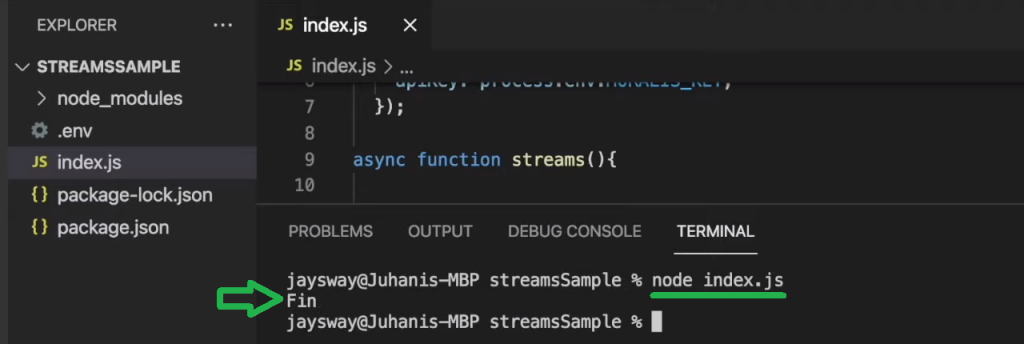
Native Foreign money Instance Transaction
Earlier than you go and execute a local foreign money transaction for testing functions, open your “Categorical server” terminal. There, you need to see an empty webhook initializing:
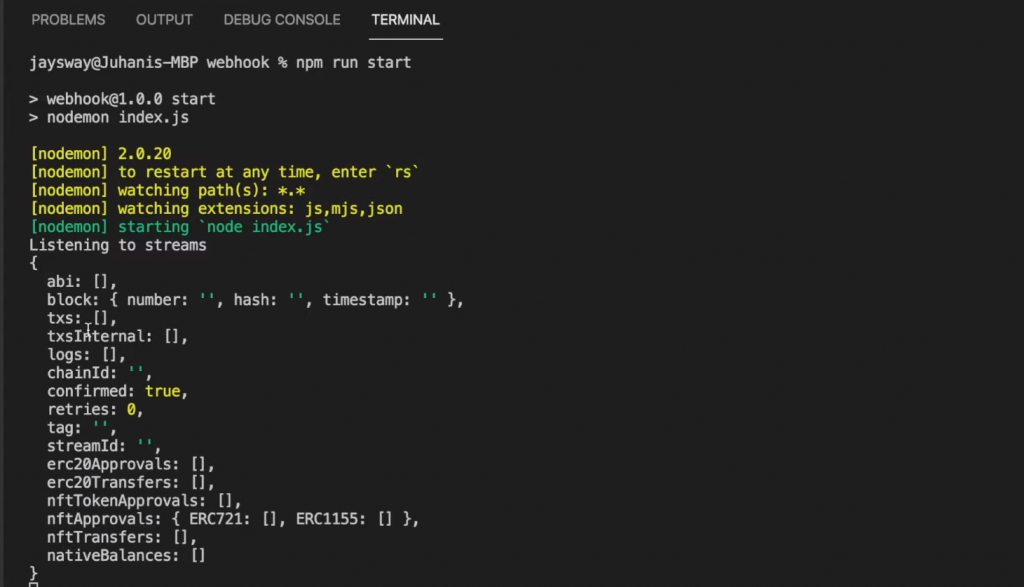
Now, use your MetaMask and switch some “take a look at” MATIC from or to the tackle you’re monitoring. As soon as the transaction is confirmed, you’ll have the ability to see the small print of your take a look at transaction in your terminal:

And that’s the best way to monitor all ETH switch transactions utilizing Moralis Streams through its SDK!
What’s Crypto Transaction Monitoring?
Whether or not you resolve to trace particular sensible contracts or wallets, all of it comes all the way down to listening to real-time, on-chain transactions. And since transactions revolve round completely different actions, you might have many choices.
As an example, within the above tutorial, we first targeted on ERC-20 transfers after which on native transfers. Nevertheless, these are simply two well-liked examples of on-chain occasions. There are a number of different on-chain occasions you may concentrate on. And all types of dapps can drastically profit from utilizing this type of monitoring.
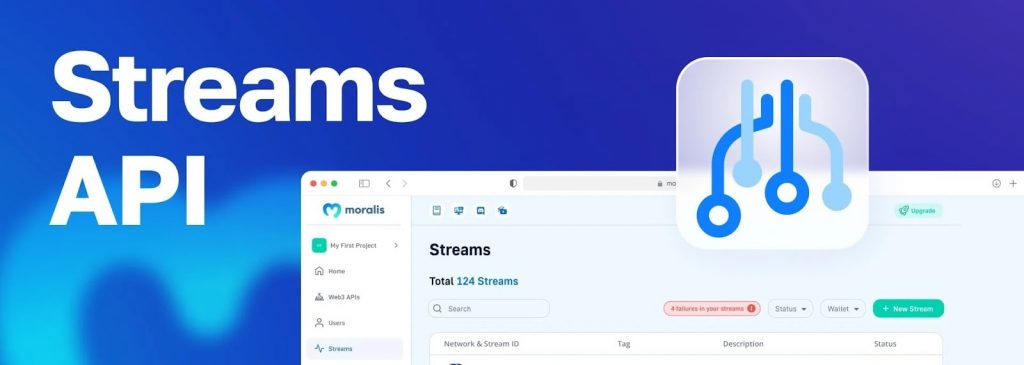
Greatest Crypto Transaction Monitoring Instrument
As you most likely know, on-chain knowledge (for public blockchains) is accessible to anybody. Nevertheless, in its uncooked format, this knowledge isn’t helpful. So, devs can both waste their time and sources by parsing the information themselves, or they will use wonderful instruments to do the work for them.
Relating to monitoring crypto transactions, Moralis’ Streams API is the very best device. It permits devs to detect occasions as they happen, and it returns parsed knowledge associated to these occasions. Consequently, this protects dapp builders a ton of time and permits them to concentrate on creating the very best UX as a substitute.
A number of the core advantages of the Streams API device embrace:
✅ Cross-chain interoperability ✅ Consumer-friendliness (cross-platform interoperability) ✅ Listening to crypto wallets and sensible contracts ✅ Pace and effectivity ✅ Superior filtering choices ✅ Accessible with a free account
What’s extra, with this highly effective device in your nook, you additionally keep away from:
❌ Connecting and sustaining buggy RPC nodes ❌ Constructing pointless abstractions ❌ Losing time constructing complicated knowledge pipelines
There are some devs who’re nonetheless utilizing ethers.js to hearken to the blockchain and, in flip, miss out on the entire above advantages. If you’re certainly one of them, ensure that to take a look at our ethers.js vs Web3 streams comparability.

The way to Monitor All ETH Switch Transactions – Abstract
In at present’s article, you had an opportunity to tackle our instance tutorial. Moreover, we demonstrated two completely different paths to finish the feat relating to the best way to monitor ETH switch transactions, together with utilizing Moralis’ admin UI or SDK.
Within the first instance, we targeted on monitoring ERC-20 token transactions. Nevertheless, within the SDK instance, we targeted on native currencies, which is the choice you’d want to decide on when specializing in ETH transfers. In each examples, we focused Polygon’s testnet; nevertheless, the rules are the identical for all supported EVM-compatible chains, together with Ethereum.
So, ensure that to make use of the information obtained on this article to start out monitoring on-chain actions with out breaking a sweat or the financial institution!
Don’t neglect to maneuver past the “the best way to monitor all ETH switch transactions” problem. In any case, there are lots of different options you may incorporate into your killer dapps. Fortuitously, for a lot of Web3 options, you may keep away from the entice of reinventing the wheel through the use of Moralis’ Web3 API fleet. The NFT API, Market Information API, Token API, Worth API, and Auth API are some well-liked examples. Furthermore, if you wish to study extra about these highly effective shortcuts, ensure that to go to Moralis’ homepage and discover the “Merchandise” menu choice.
Then again, it’s possible you’ll be new to crypto and have to study extra about dapp growth first. In that case, we suggest diving into Moralis’ weblog. A number of the newest subjects there’ll allow you to find out about NFT market growth, the getLogs technique, Web3 market knowledge API, and rather more. Nonetheless, you could be enthusiastic about taking an expert strategy to your crypto training. In that case, contemplate enrolling in Moralis Academy.