In at this time’s tutorial, we’ll present you learn how to checklist all of the cash in an ETH handle utilizing the Moralis Token API – the {industry}’s main software for ERC-20 knowledge. Because of the accessibility of the Token API, you may get all of the cash of any handle with a single name to the getWalletTokenBalances() endpoint. Right here’s a bit sneak peek of what the code would possibly appear like:
const Moralis = require(“moralis”).default;
const { EvmChain } = require(“@moralisweb3/common-evm-utils”);
const runApp = async () => {
await Moralis.begin({
apiKey: “YOUR_API_KEY”,
// …and every other configuration
});
const handle = “0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d”;
const chain = EvmChain.ETHEREUM;
const response = await Moralis.EvmApi.token.getWalletTokenBalances({
handle,
chain,
});
console.log(response.toJSON());
};
runApp();
You merely have so as to add your Moralis API key, configure handle to suit your question and run the code. In return, you’ll get a response containing a listing of all of the cash from the desired ETH handle.
That’s it; it doesn’t need to be tougher than that when working with Moralis. Nonetheless, if you’d like a extra detailed breakdown of your complete course of, be a part of us on this tutorial or take a look at the Moralis documentation web page on learn how to get all tokens owned by an handle!
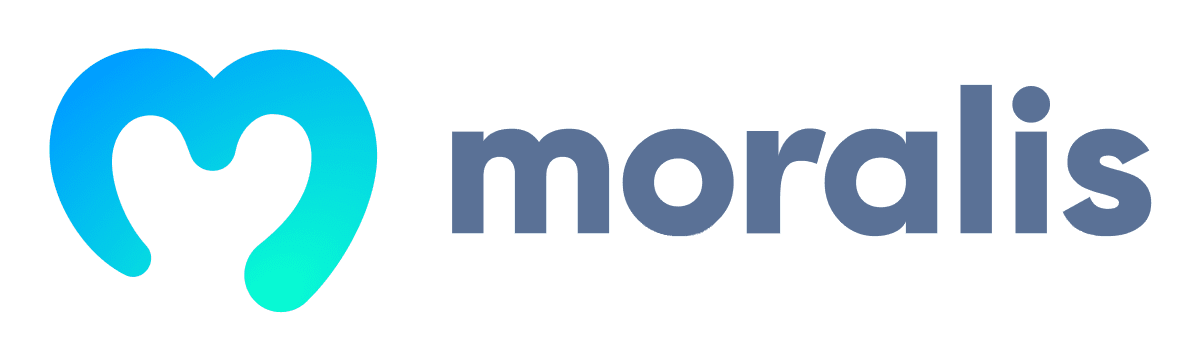
Additionally, if you happen to want to name the Token API your self, don’t overlook to enroll with Moralis. You possibly can arrange your account with out paying a dime, and also you’ll get immediate entry to all our industry-leading Web3 APIs!
Overview
We’ll kickstart at this time’s article by diving into the ins and outs of ETH addresses. In doing so, we’ll cowl what they’re and what they’re used for. From there, we’ll soar straight into the tutorial and present you learn how to checklist all of the cash in an ETH handle in three easy steps utilizing Moralis’ Token API:
Get a Moralis API KeyWrite a Script Calling the getWalletTokenBalances() EndpointRun the Code
From there, we’ll discover some outstanding use circumstances for itemizing all of the cash in an ETH handle earlier than we high issues off by diving deeper into Moralis!
Now, with out additional ado, let’s get going and discover the intricacies of ETH addresses!
What’s an ETH Deal with?
An ETH handle is a 42-character lengthy, alphanumerical hex string serving as a singular and public identifier for an externally owned account (EOA) or sensible contract on the Ethereum blockchain. Such an handle is usually used for sending, receiving, and storing digital belongings like fungible and non-fungible tokens (NFTs)!
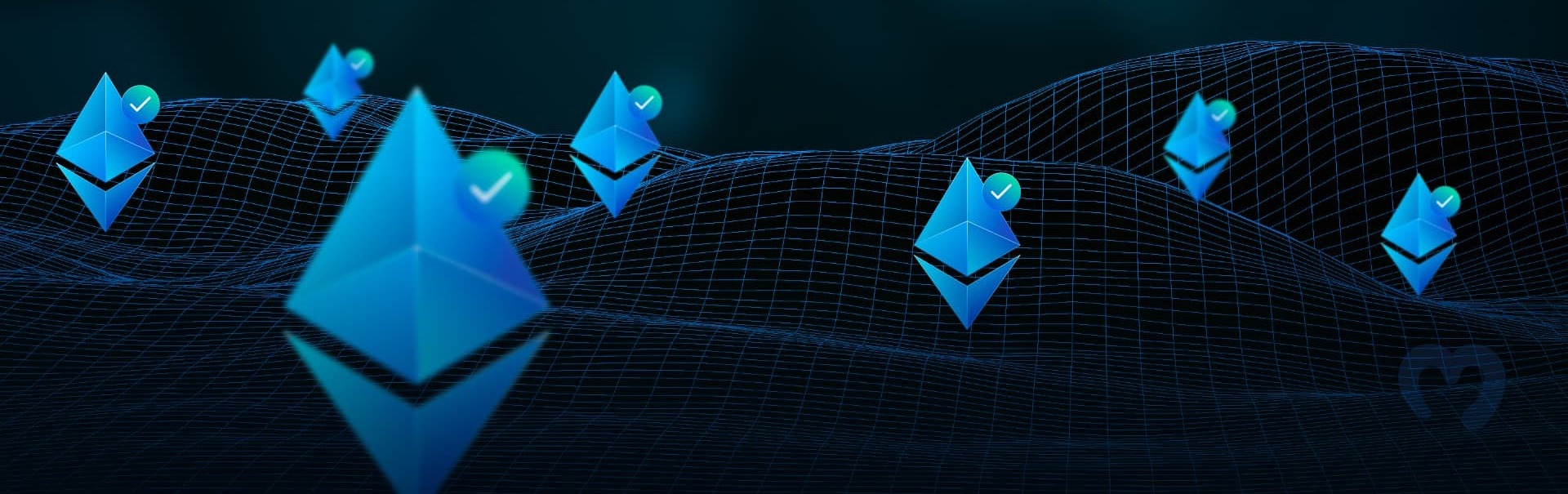
There are two varieties of ETH addresses, and we’ll briefly cowl each beneath:
Account Addresses: An account handle – additionally generally known as a ”pockets handle” or ”blockchain handle” – corresponds to a public person account on the blockchain managed by a non-public key. Account addresses are used to ship, obtain, and retailer digital belongings, together with interacting with sensible contracts and dapps. Contract Addresses: Contract addresses are related to sensible contracts deployed on the blockchain. A contract handle isn’t managed by a person or entity like an account handle however quite represents the placement the place the sensible contract resides on the community.
All in all, ETH addresses are distinctive identifiers for Ethereum accounts and sensible contracts. Moreover, they’re generally used for sending, receiving, and storing digital belongings like fungible tokens and NFTs!
Since ETH addresses are public, we will use them to search for details about accounts and sensible contracts. This contains issues corresponding to token balances, which we’ll present you how one can fetch within the following part utilizing Moralis!
Full Tutorial: Checklist All of the Cash in An ETH Deal with
The simplest option to checklist all of the cash in an ETH handle is to leverage Moralis’ Token API – the last word interface for real-time ERC-20 knowledge. The truth is, because of the accessibility of this industry-leading software, you may get all the knowledge you want straight from the Ethereum blockchain in three easy steps:
Get a Moralis API KeyWrite a Script Calling the getWalletTokenBalances() EndpointRun the Code
Nonetheless, earlier than leaping into the preliminary step of this tutorial, you will need to first maintain a few conditions!
Conditions
Moralis’ Web3 APIs are appropriate with a number of programming languages, together with Python, TypeScript, and many others. Nonetheless, for this tutorial, we’ll be sticking to JavaScript. As such, earlier than you proceed, please ensure you have the next prepared:
Step 1: Get a Moralis API Key
To name the Token API, you want a Moralis API key, which you may get totally free by signing up with Moralis. As such, if you happen to haven’t already, click on on the ”Begin for Free” button on the high proper of the Moralis homepage to create your account:
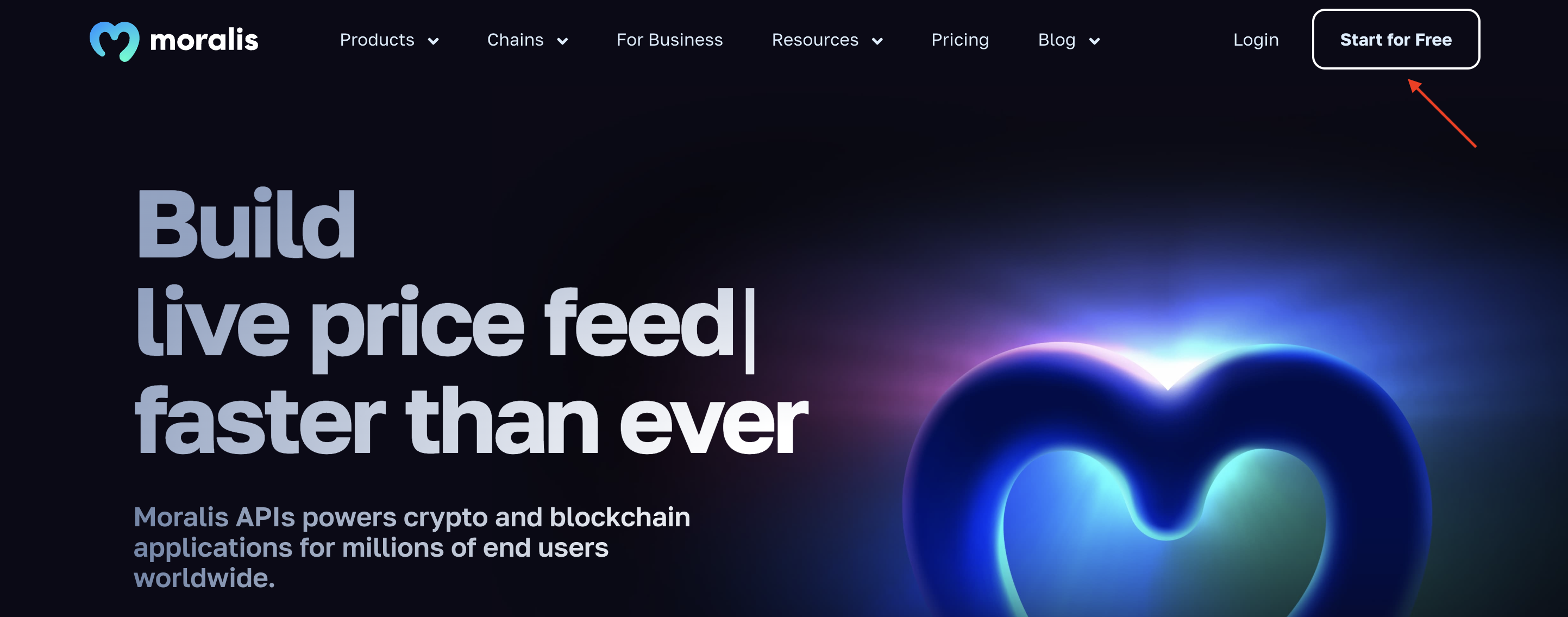
With an account at hand, choose a undertaking, go to the ”Settings” tab utilizing the navigation bar to the left, scroll right down to the ”API Keys” part, and duplicate your key:
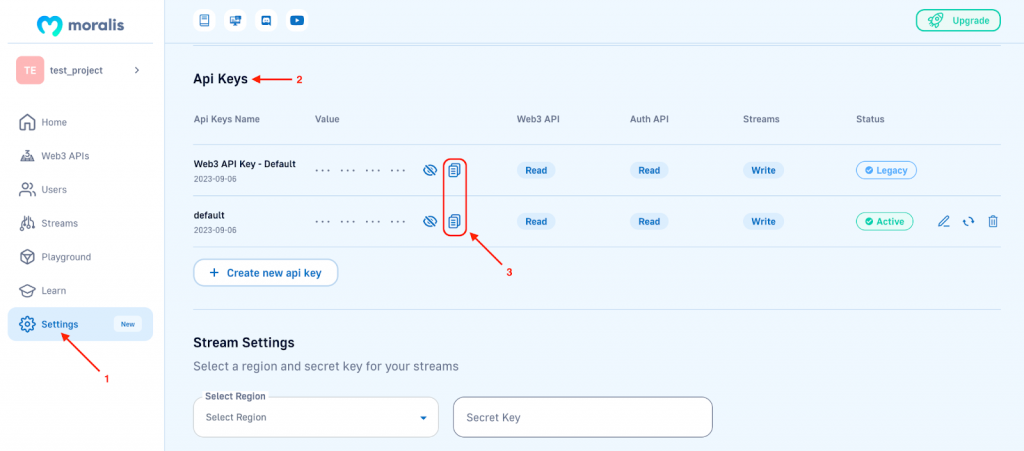
Save the important thing for now, as you’ll want it within the subsequent step when initializing the Moralis software program improvement package (SDK)!
Step 2: Write a Script Calling the getWalletTokenBalances() Endpoint
For the second step, begin by establishing a brand new undertaking folder in your most popular IDE. From there, open a brand new terminal and run the next command within the root folder to put in the Moralis SDK:
npm set up moralis @moralisweb3/common-evm-utils
Subsequent, create a brand new ”index.js” file and add the next code:
const Moralis = require(“moralis”).default;
const { EvmChain } = require(“@moralisweb3/common-evm-utils”);
const runApp = async () => {
await Moralis.begin({
apiKey: “YOUR_API_KEY”,
// …and every other configuration
});
const handle = “0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d”;
const chain = EvmChain.ETHEREUM;
const response = await Moralis.EvmApi.token.getWalletTokenBalances({
handle,
chain,
});
console.log(response.toJSON());
};
runApp();
Earlier than working the code, that you must make just a few minor configurations. Begin by changing YOUR_API_KEY with the important thing you copied throughout step one. Doing so will initialize the Moralis SDK:
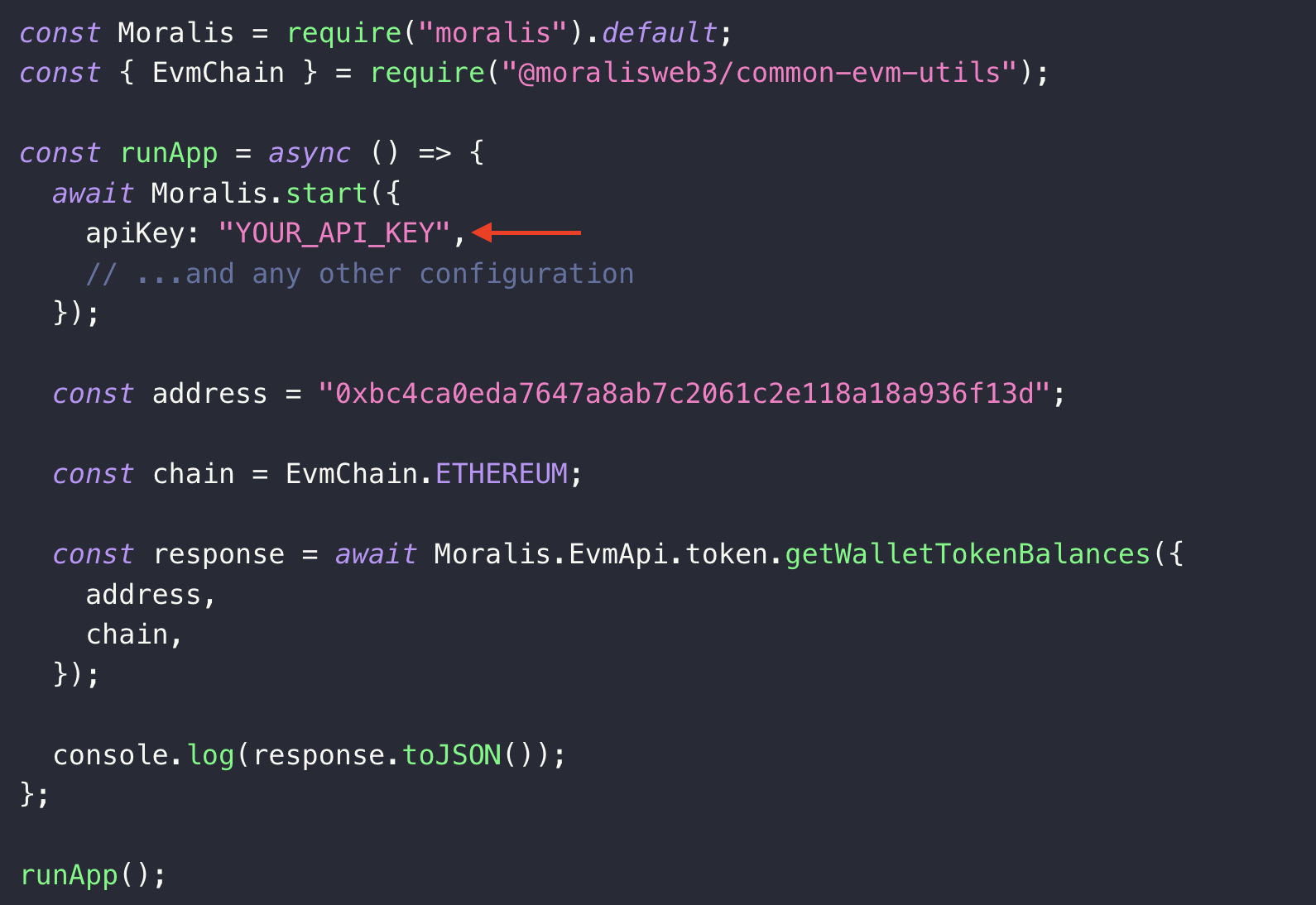
Understand that you don’t need to expose your Moralis API key. As such, when constructing production-ready dapps, make certain to make use of setting variables and different greatest practices.
From right here, you additionally must configure the handle const by including the ETH handle from which you need to get the checklist of all cash:
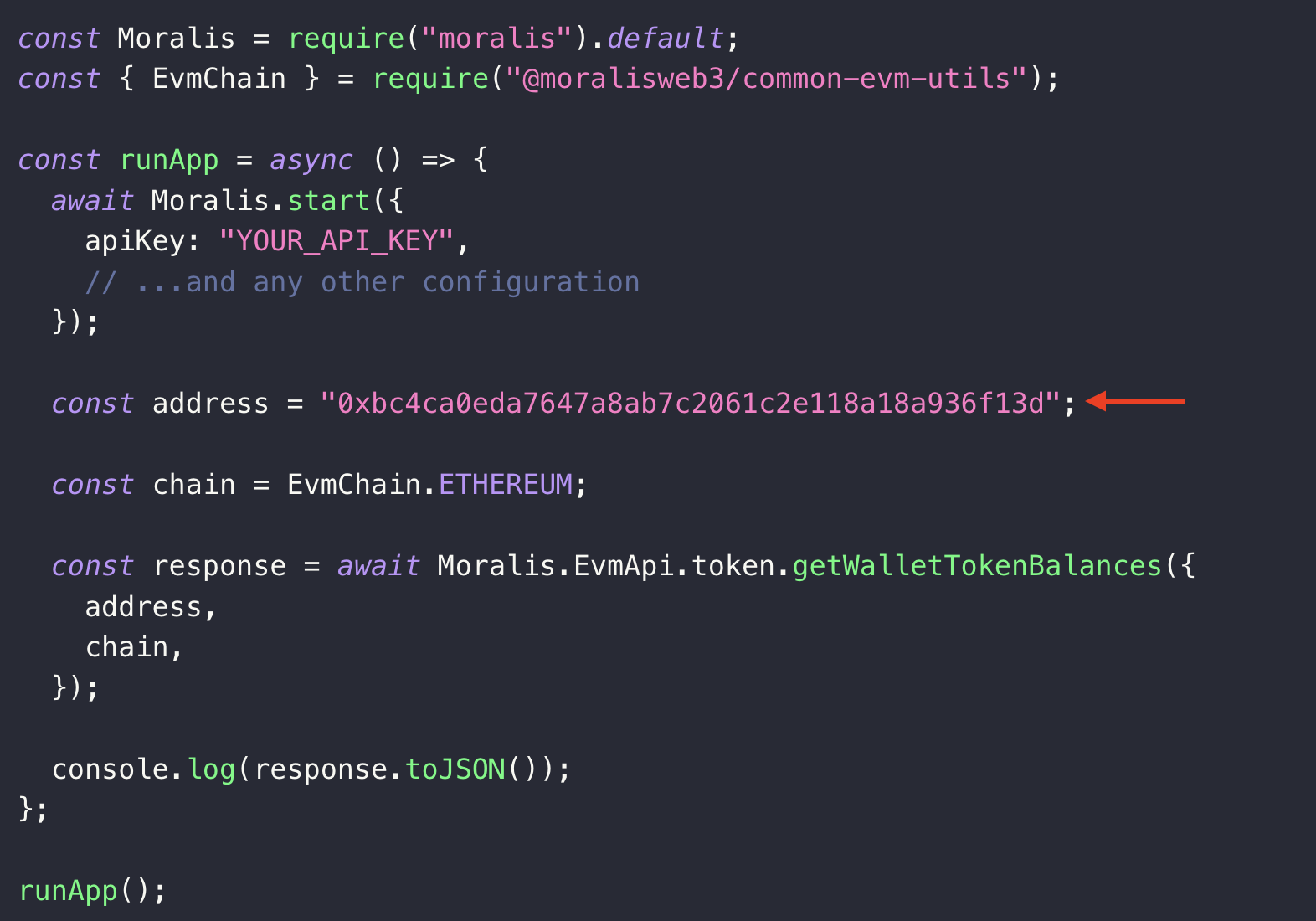
We then go alongside handle and chain as parameters when calling the getWalletTokenBalances() endpoint:
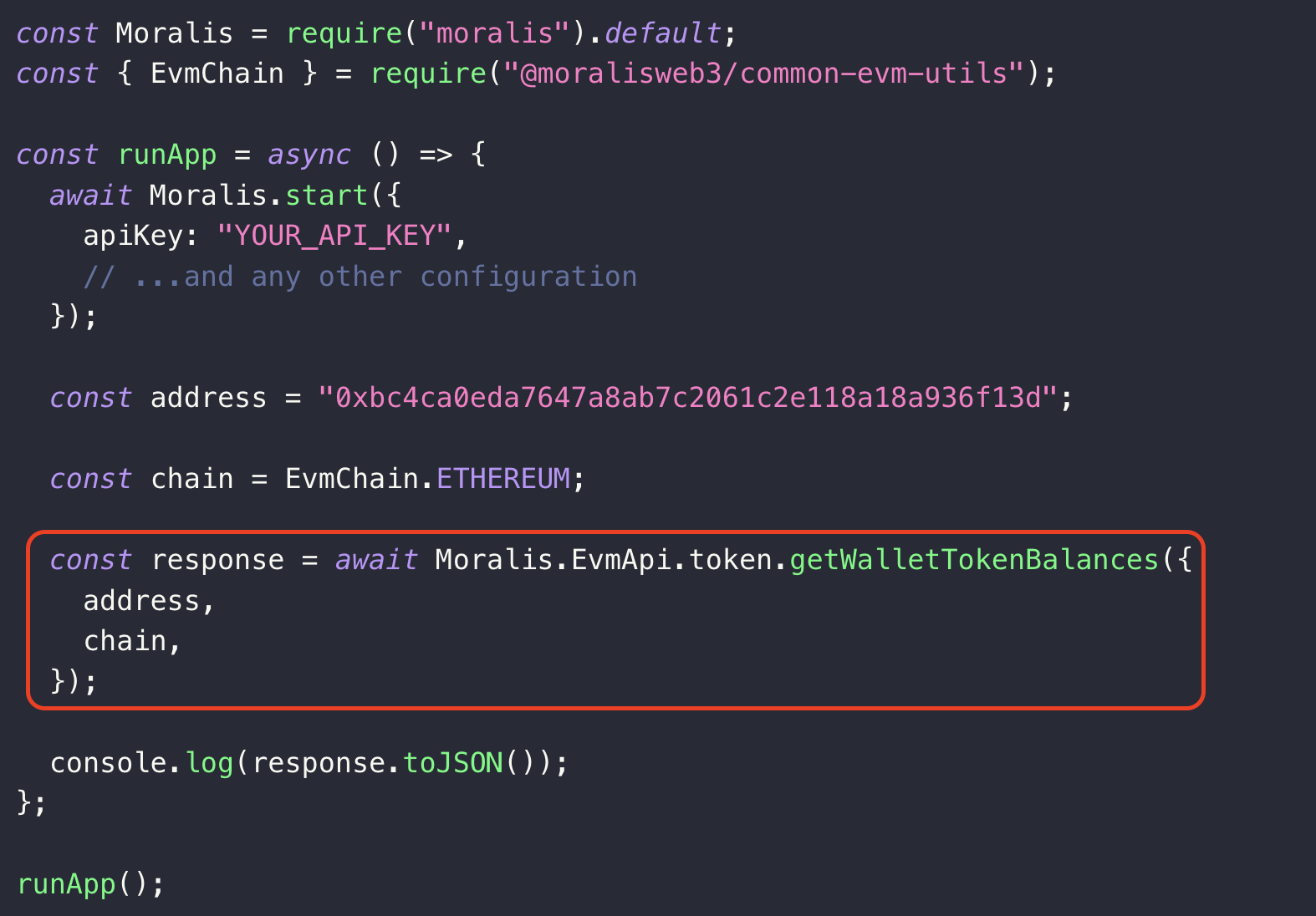
That’s it for the code; you’re now able to run the script and fetch a listing of all of the cash within the specified ETH handle!
Step 3: Run the Code
To run the code, go forward and launch a brand new terminal, cd into the undertaking’s root folder, and execute the next command:
node index.js
When you run the code, you’ll get a response containing a listing of all of the cash within the specified ETH handle. It ought to look one thing like this:
[
{
“token_address”: “0xff20548405c6f024264b5c8dc954625285b799e1”,
“symbol”: “BTCETF”,
“name”: “BTCETF”,
“logo”: null,
“thumbnail”: null,
“decimals”: 18,
“balance”: “166000000000000000000000”,
“possible_spam”: false
},
{
“token_address”: “0xe90cc7d807712b2b41632f3900c8bd19bdc502b1”,
“symbol”: “KUMA”,
“name”: “Kuma”,
“logo”: null,
“thumbnail”: null,
“decimals”: 18,
“balance”: “3000000000000000000000000000”,
“possible_spam”: false
},
//…
]
Congratulations; you now know learn how to get a listing of all of the cash in an ETH handle!
Now, if you’d like inspiration on what to do with this knowledge, be a part of us within the following part. In doing so, we’ll discover some widespread use circumstances for itemizing all of the cash in an ETH handle!
Use Instances for Itemizing All of the Cash in An ETH Deal with
There are various use circumstances for itemizing all of the cash in an ETH handle, and we gained’t have the ability to cowl all of them on this part. As an alternative, we’ll briefly checklist 4 outstanding examples of platforms that want entry to handle balances in some capability:
Portfolio Trackers: A crypto portfolio tracker is a digital platform that permits you to oversee all of your cryptocurrency holdings in a single place. Some nice examples embody Delta, Koinly, and Kubera. Decentralized Exchanges (DEXs): A DEX is a crypto change facilitating peer-to-peer (P2P) transactions with out middleman involvement. Examples of well-established platforms embody Uniswap, Curve Finance, and PancakeSwap. Block Explorers: A block explorer is a digital software that permits you to seek for real-time and historic details about a blockchain community. This contains all the pieces from block knowledge to token balances of a selected handle. Some outstanding examples of block explorers embody Etherscan, PolygonScan, and BscScan. Web3 Wallets: Web3 wallets are digital platforms that assist you to retailer and handle your digital belongings, together with shopping for, promoting, and swapping tokens. Examples of Web3 wallets are MetaMask, Coinbase Pockets, and Ledger.
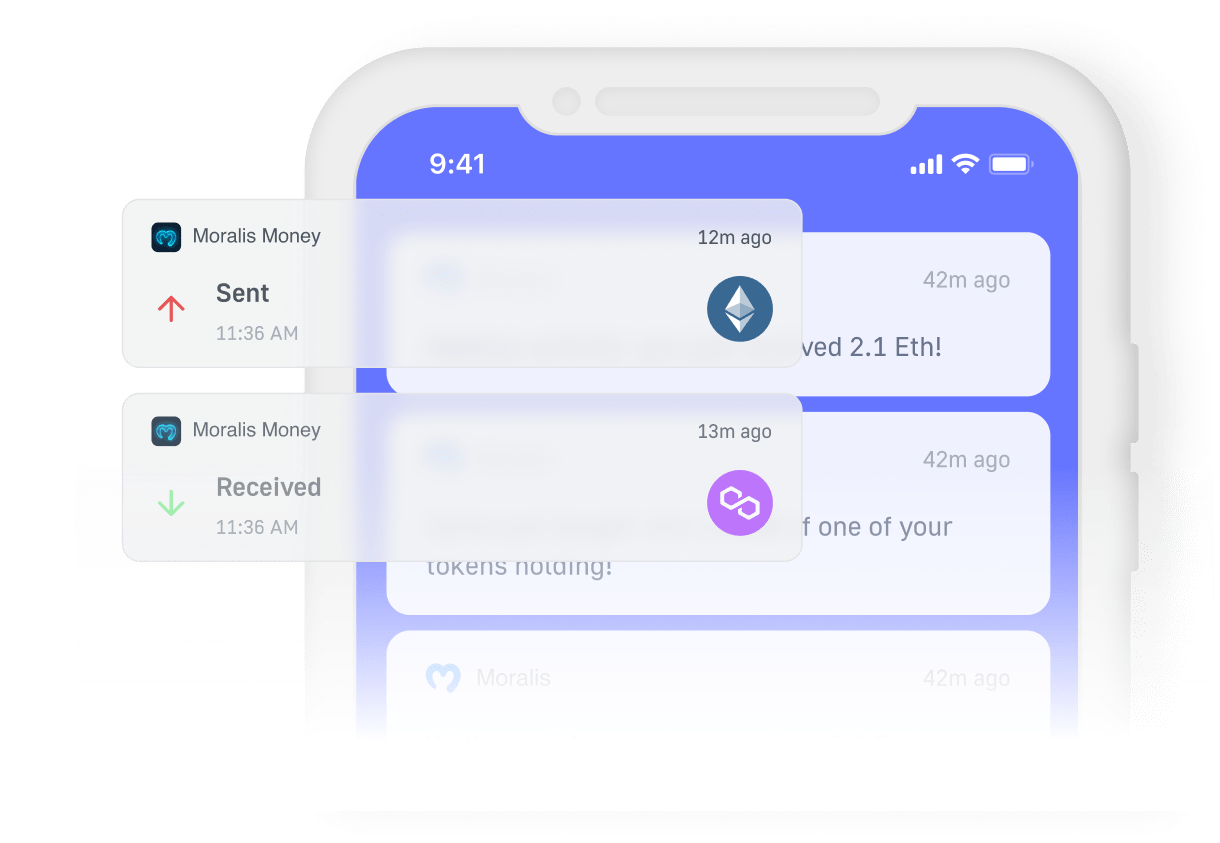
Now that you understand how to checklist all of the cash in an ETH handle and a few situations when this knowledge may be useful, let’s discover Moralis’ capabilities additional within the subsequent part!
Past Querying a Checklist of All of the Cash in An ETH Deal with – Exploring Moralis Additional
Moralis is the {industry}’s premier Web3 API supplier, and the capabilities of our dynamic toolset prolong far past the Token API!
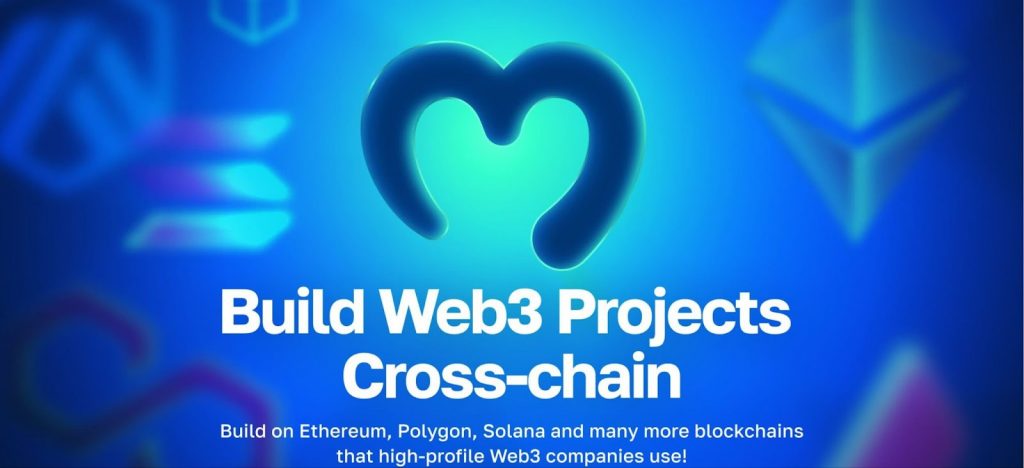
In Moralis’ industry-leading Web3 API suite, you’ll discover a number of interfaces for varied use circumstances, and down beneath, we’ll discover three examples:
Pockets API: Our Pockets API is the last word software if you happen to’re trying to construct Web3 wallets or combine pockets performance into your dapps. With single API calls, you possibly can effortlessly fetch pockets balances, real-time transfers, profile knowledge, and far more. As an example, right here’s an instance of how straightforward it’s to fetch the native steadiness of any pockets with the getNativeBalance() endpoint: const response = await Moralis.EvmApi.steadiness.getNativeBalance({
“chain”: “0x1”,
“handle”: “0xDC24316b9AE028F1497c275EB9192a3Ea0f67022”
}); NFT API: Moralis’ NFT API is the {industry}’s main software for NFT-related knowledge. With this premier interface, you may get NFT metadata, balances, on-chain costs, and extra with solely single traces of code. For instance, with the getWalletNFTs() endpoint, you possibly can seamlessly fetch the NFT steadiness of any pockets: const response = await Moralis.EvmApi.nft.getWalletNFTs({
“chain”: “0x1”,
“handle”: “0xff3879b8a363aed92a6eaba8f61f1a96a9ec3c1e”
}); Blockchain API: With the Blockchain API, you possibly can unlock the ability of uncooked and decoded blockchain knowledge. Fetch block knowledge, sensible contract logs, occasions, and far more with ease. As an example, right here’s an instance of how one can get block knowledge – together with hashes, timestamps, and many others. – with the getBlock() endpoint: const response = await Moralis.EvmApi.block.getBlock({
“chain”: “0x1”,
“blockNumberOrHash”: “18541416”
});
So, it doesn’t matter if you happen to’re constructing Web3 wallets, NFT dapps, blockchain explorers, or every other platform; Moralis has the proper instruments in your wants. Should you’d wish to discover all our premier interfaces, take a look at our official Web3 API web page!
Nonetheless, at this level, you would possibly nonetheless be asking your self, ”Why ought to I leverage Moralis’ Web3 APIs?”. To reply this query, let’s discover some outstanding advantages of Moralis within the following subsection!
Advantages of Moralis’ Web3 APIs
Moreover permitting you to construct Web3 tasks quicker and extra effectively, Moralis supplies many benefits. Nonetheless, sadly, we will be unable to cowl all the advantages on this article. As an alternative, now we have narrowed it down to 3 outstanding examples of why it’s best to use Moralis:
High Efficiency: There are various good Web3 API suppliers in the marketplace; nevertheless, Moralis stands out as the very best in the case of efficiency. Whether or not you need to measure by reliability, velocity, protection, or every other metric, Moralis all the time comes out on high. Unparalleled Scalability: Moralis’ APIs supply unparalleled scalability. Consequently, our APIs will scale with out fault as your person base grows. Cross-Chain Compatibility: Our Web3 APIs are chain agnostic, supporting all main blockchain networks. This contains Ethereum, BNB Sensible Chain, Solana, and many others., together with a number of outstanding ETH layer-2 scaling options. As such, when working with Moralis, you’ll don’t have any bother constructing cross-chain appropriate dapps!
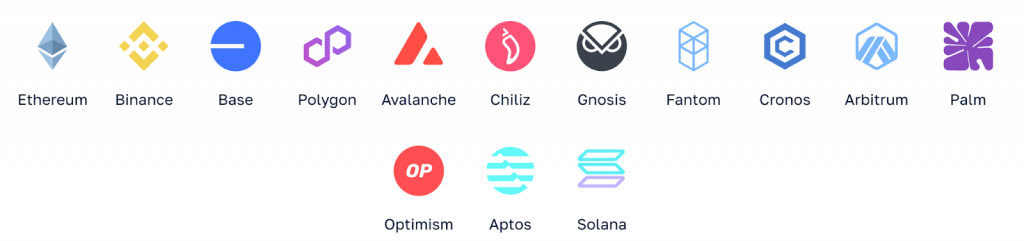
So, if you happen to’re severe about constructing Web3 tasks, make certain to enroll with Moralis. You possibly can create your account totally free, and also you’ll achieve immediate entry to all our industry-leading Web3 improvement instruments!
Abstract: How you can Checklist All of the Cash in An ETH Deal with
In at this time’s article, we kicked issues off by diving into the ins and outs of ETH addresses. In doing so, we discovered that they function distinctive identifiers for EOAs and sensible contracts on the Ethereum blockchain.
From there, we then jumped straight into the primary tutorial and confirmed you learn how to checklist all of the cash in an ETH handle in three steps utilizing the Moralis Token API:
Get a Moralis API KeyWrite a Script Calling the getWalletTokenBalances() EndpointRun the Code
So, when you’ve got adopted alongside this far, you now know learn how to fetch all of the tokens of Ethereum addresses!
We then additionally explored some outstanding use circumstances for this knowledge. In doing so, we briefly coated Web3 wallets, portfolio trackers, DEXs, and block explorers. Should you preferred this tutorial on learn how to checklist all of the cash in an ETH handle, take into account studying extra content material right here on the Moralis weblog. For instance, take a look at our most up-to-date Starknet faucet information or discover the ins and outs of Web3 social media.
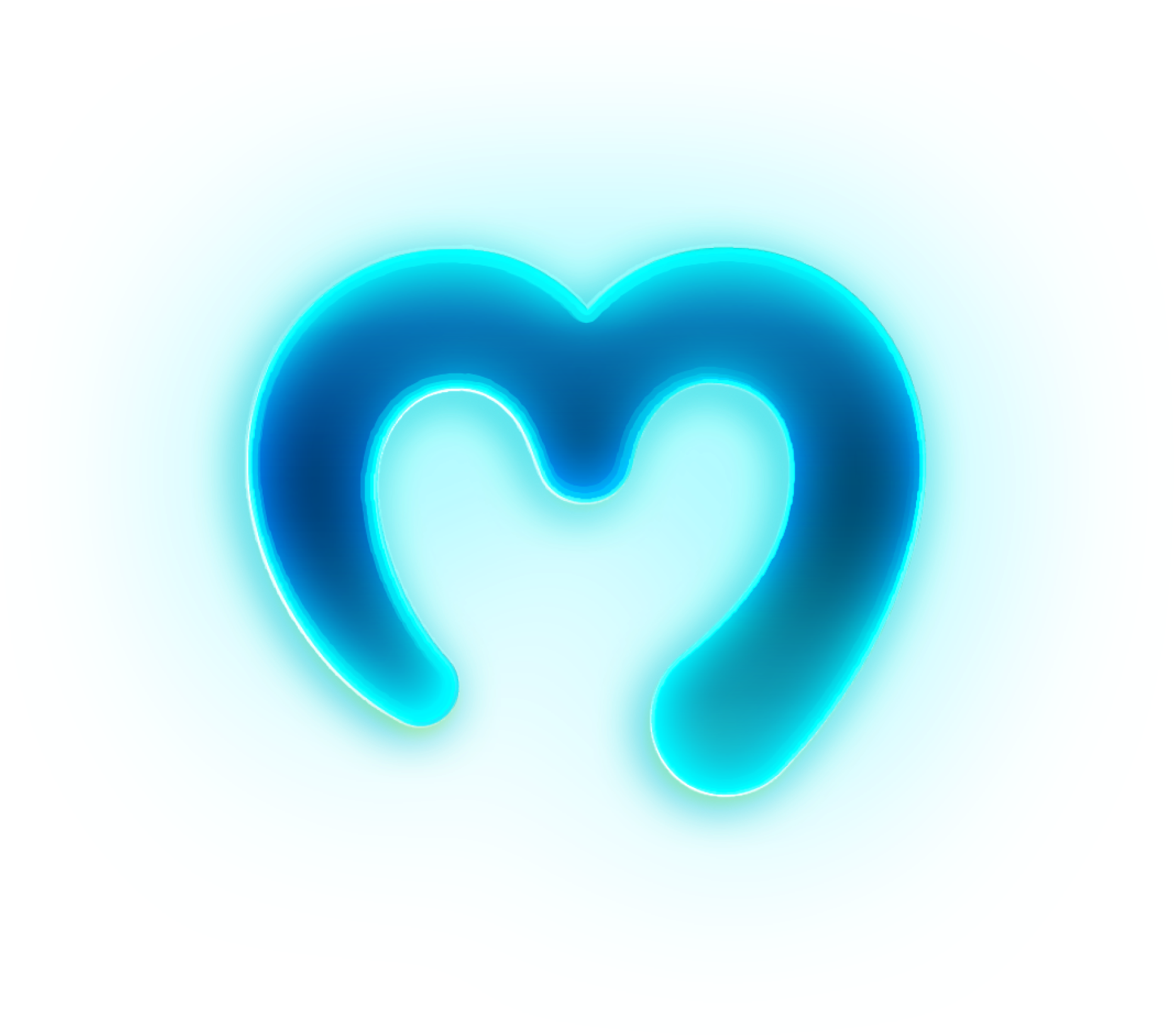
Additionally, if you wish to leverage our Web3 APIs in your improvement endeavors, don’t overlook to enroll with Moralis. You possibly can arrange your account totally free, and also you’ll have the ability to begin constructing dapps quicker and smarter at this time!