On this article, we’ll present you how one can create a token on BNB Good Chain (BSC) utilizing Remix, breaking down the method into 5 easy steps. However if you happen to’re wanting to dive straight into the code of our token contract, you’ll find it right here:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC20/ERC20.sol”;
contract BEP20 is ERC20 {
constructor(uint256 initialSupply) ERC20(“BEP20Test”, “BPT”) {
_mint(msg.sender, initialSupply);
}
}
For a extra complete breakdown of the code above, together with detailed directions on compiling and deploying the contract utilizing Remix, we invite you to hitch us on this information, providing a extra in-depth tutorial on how one can create a token on BSC!
Overview
We’ll kickstart right now’s article by diving into the ins and outs of BSC tokens. From there, we’ll discover Remix IDE and MetaMask, as we’ll use each these instruments to jot down and deploy our BSC token contract. Subsequent, we’ll bounce straight into our tutorial to indicate you how one can create a BSC token with Remix in 5 simple steps:
Add the BSC Testnet to MetaMaskGet BSC Testnet TokensCreate Your BSC Token Contract with RemixDeploy Your ContractAdd the Token to MetaMask
Lastly, for these additional keen on Web3 growth, we’ll additionally introduce you to Moralis – the {industry}’s main Web3 API supplier!
In Moralis’ suite of Web3 APIs, you’ll discover a number of interfaces for numerous use instances. Some distinguished examples embody the NFT API, Pockets API, and plenty of others. With these instruments, you may seamlessly question and combine on-chain knowledge and blockchain performance into all tasks with only some traces of code, making Web3 growth a breeze!
Additionally, do you know you may enroll with Moralis at no cost? As such, please take this chance to supercharge your Web3 growth endeavors with our premier Web3 APIs!
However, let’s begin this text by answering the query, ”What’s a BSC token?”
What’s a BSC Token?
A BSC token is a digital asset constructed on BNB Good Chain (BSC) – an EVM-compatible blockchain community designed for operating good contract-based purposes. Furthermore, BSC tokens adhere to the BEP-20 token customary, which defines a algorithm and tips for creating and managing fungible tokens on the community!
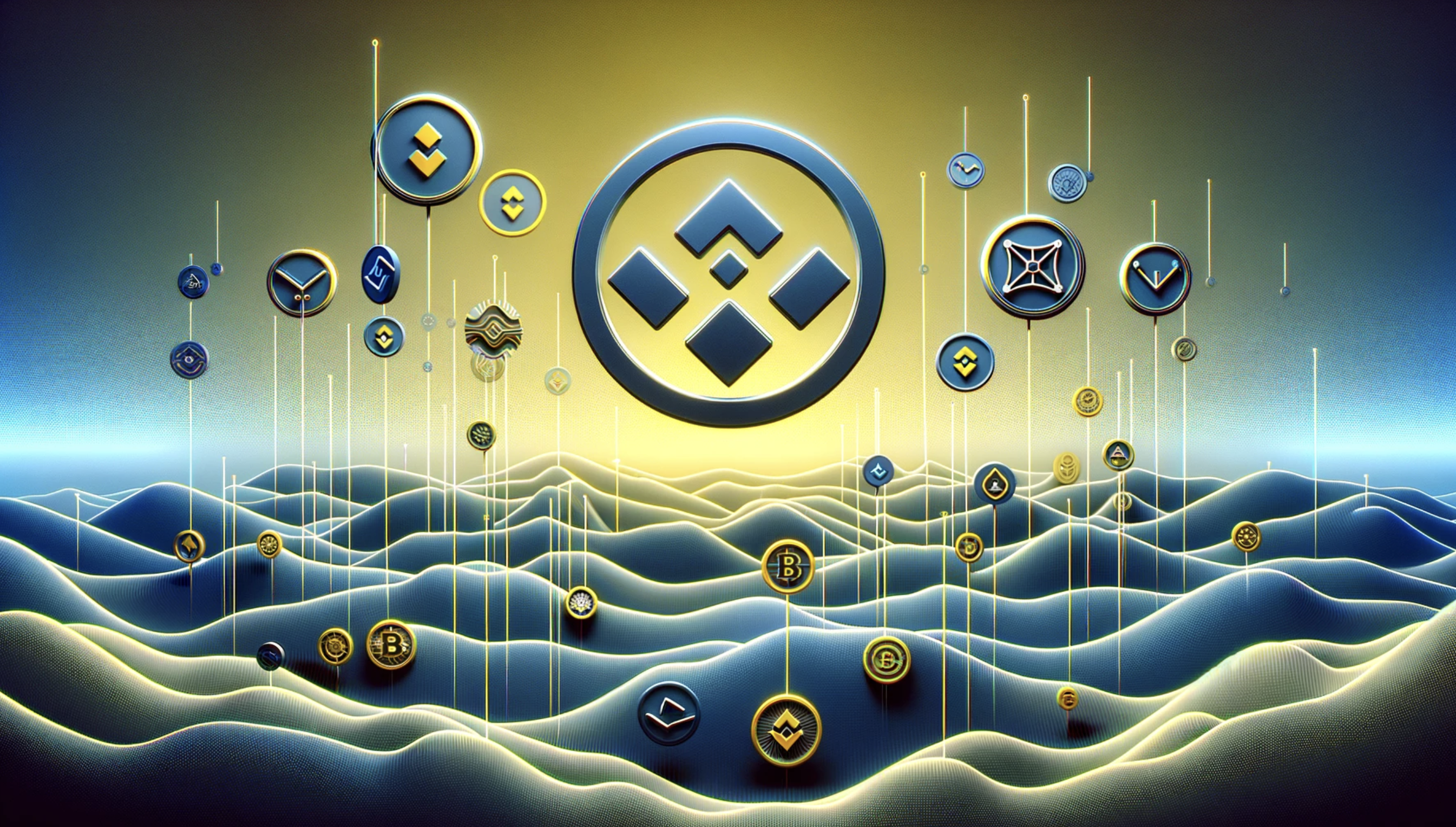
BEP-20 is an extension of Ethereum’s extensively adopted ERC-20 customary, and they’re very related. You’ll be able to consider the usual as a blueprint that defines particular capabilities and variables token contracts should implement to be thought of BEP-20 compliant.
By offering a standard customary, BEP-20 ensures consistency and interoperability amongst tokens, streamlining the method for builders to work with and combine these digital property into their Web3 wallets, decentralized exchanges (DEXs), and different platforms.
In essence, BSC tokens are tokens constructed on BNB Good Chain adhering to the BEP-20 token customary!
Making a Token on BSC with Remix & MetaMask
In right now’s article, we’ll present you how one can create a BSC token utilizing Remix and MetaMask. Consequently, earlier than leaping into the tutorial, let’s briefly break down each these platforms individually:
Remix IDE: Remix is an built-in growth surroundings (IDE) that includes an intuitive graphical person interface and a strong toolset for writing, testing, debugging, compiling, and deploying EVM-compatible good contracts. What’s extra, Remix affords a variety of plugins and extensions, permitting you to seamlessly tailor your growth surroundings to your wants.
All in all, Remix is a robust, intuitive, and well-used platform that has turn into an important software in Web3’s growth ecosystem!
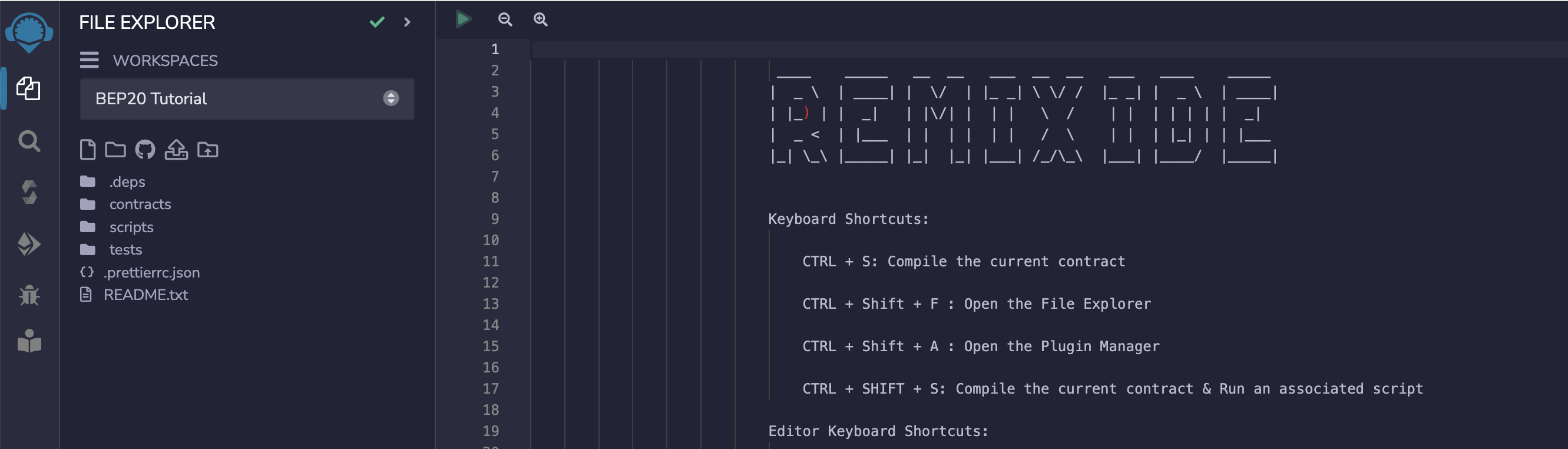
MetaMask: MetaMask is the {industry}’s main Web3 pockets, boasting a powerful 30 million month-to-month lively customers. With this EVM-compatible pockets, it’s doable to retailer, purchase, ship, and swap tokens throughout a number of chains, together with Ethereum, BSC, Polygon, and plenty of others.
Furthermore, along with permitting you to retailer and handle your digital property, MetaMask additionally acts as a gateway to Web3’s in depth ecosystem of decentralized purposes (dapps) and good contracts!
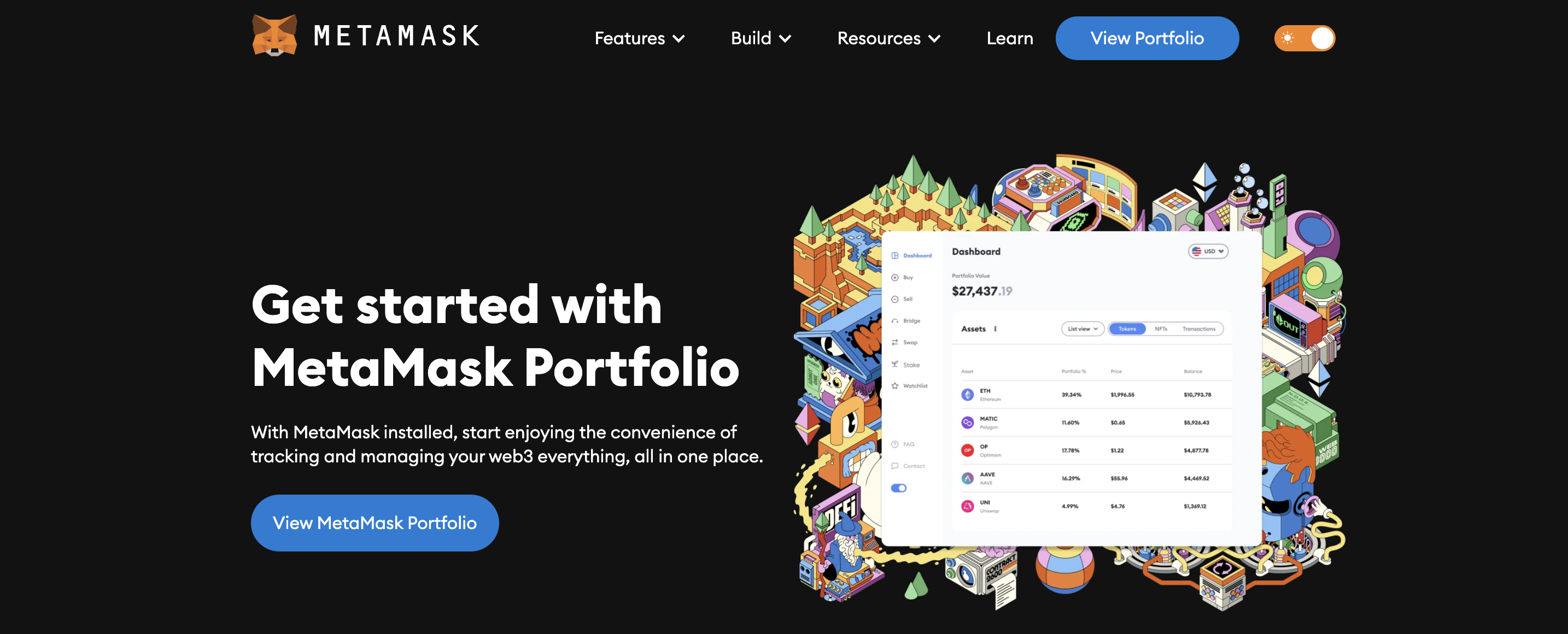
Now, with an outline of Remix and MetaMask, let’s dive straight into the principle tutorial and present you how one can create a token on BSC!
Easy methods to Create a BSC Token with Remix
Within the following subsections, we’ll present you how one can create a BSC token with Remix and MetaMask in 5 steps:
Add the BSC Testnet To MetaMaskGet BSC Testnet TokensCreate Your BSC Token Contract with RemixDeploy Your ContractAdd the Token To MetaMask
On this tutorial, we’ll make the most of the BSC testnet for comfort. Nevertheless, the process for making a token on the BSC mainnet stays almost equivalent, with the principle distinctions rising within the first and second steps, the place you have to add the mainnet and get actual BNB tokens as a substitute.
However, with out additional delay, let’s bounce into step one and present you how one can add the BSC testnet to MetaMask!
Step 1: Add the BSC Testnet To MetaMask
Including the BSC testnet to MetaMask is straightforward, and the very first thing you have to do is click on the networks drop-down menu on the high left of your MetaMask interface, adopted by ”Add community”:
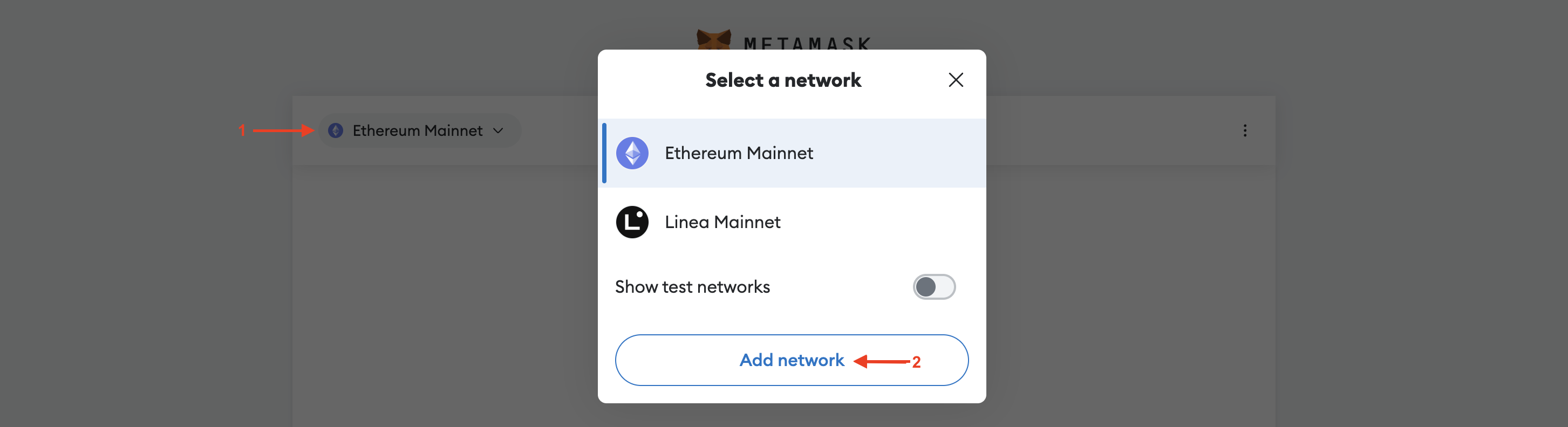
Subsequent, click on ”Add a community manually” on the backside:
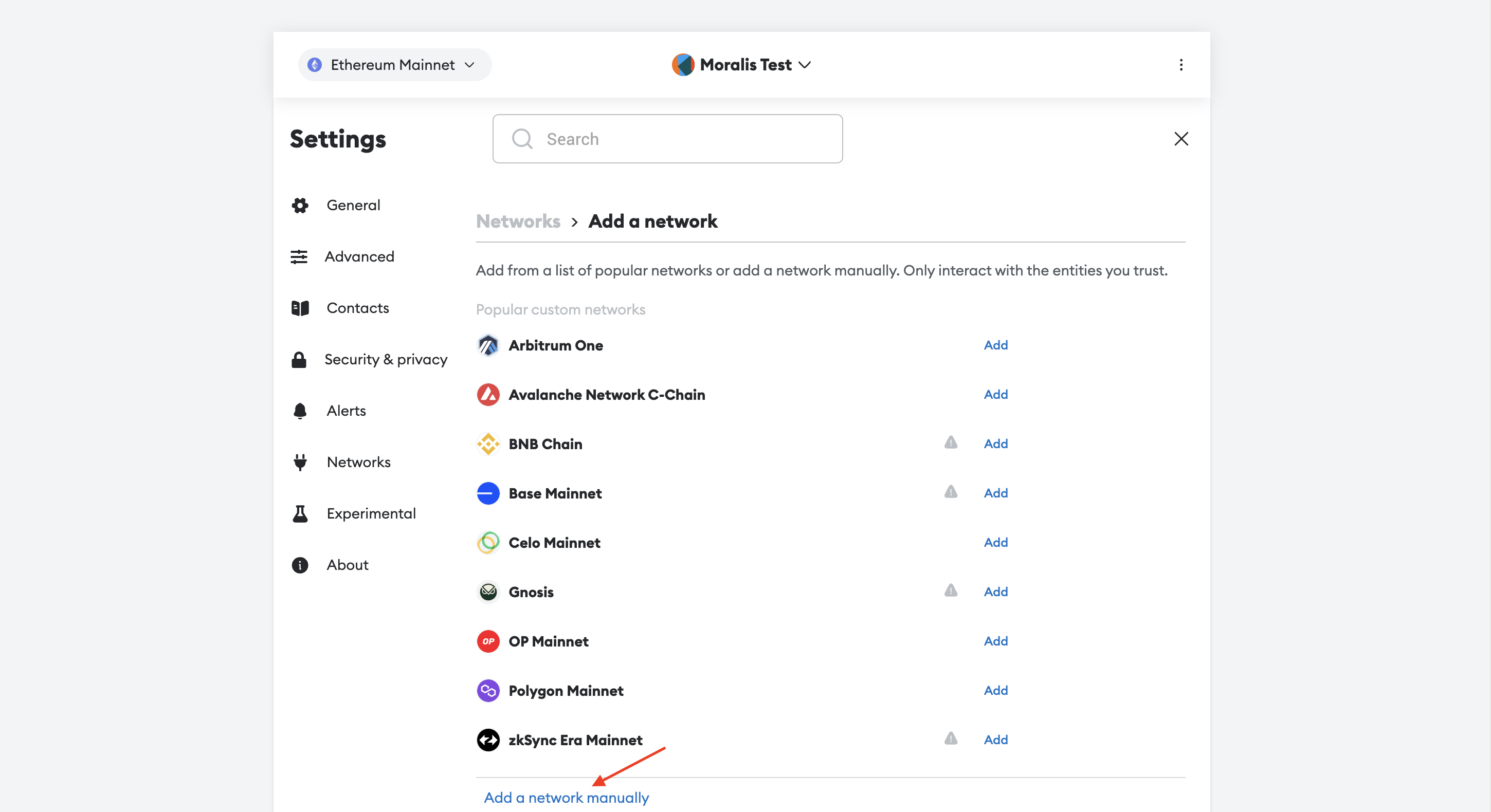
Doing so takes you to the next web page, the place you have to add the community particulars for the BSC testnet:
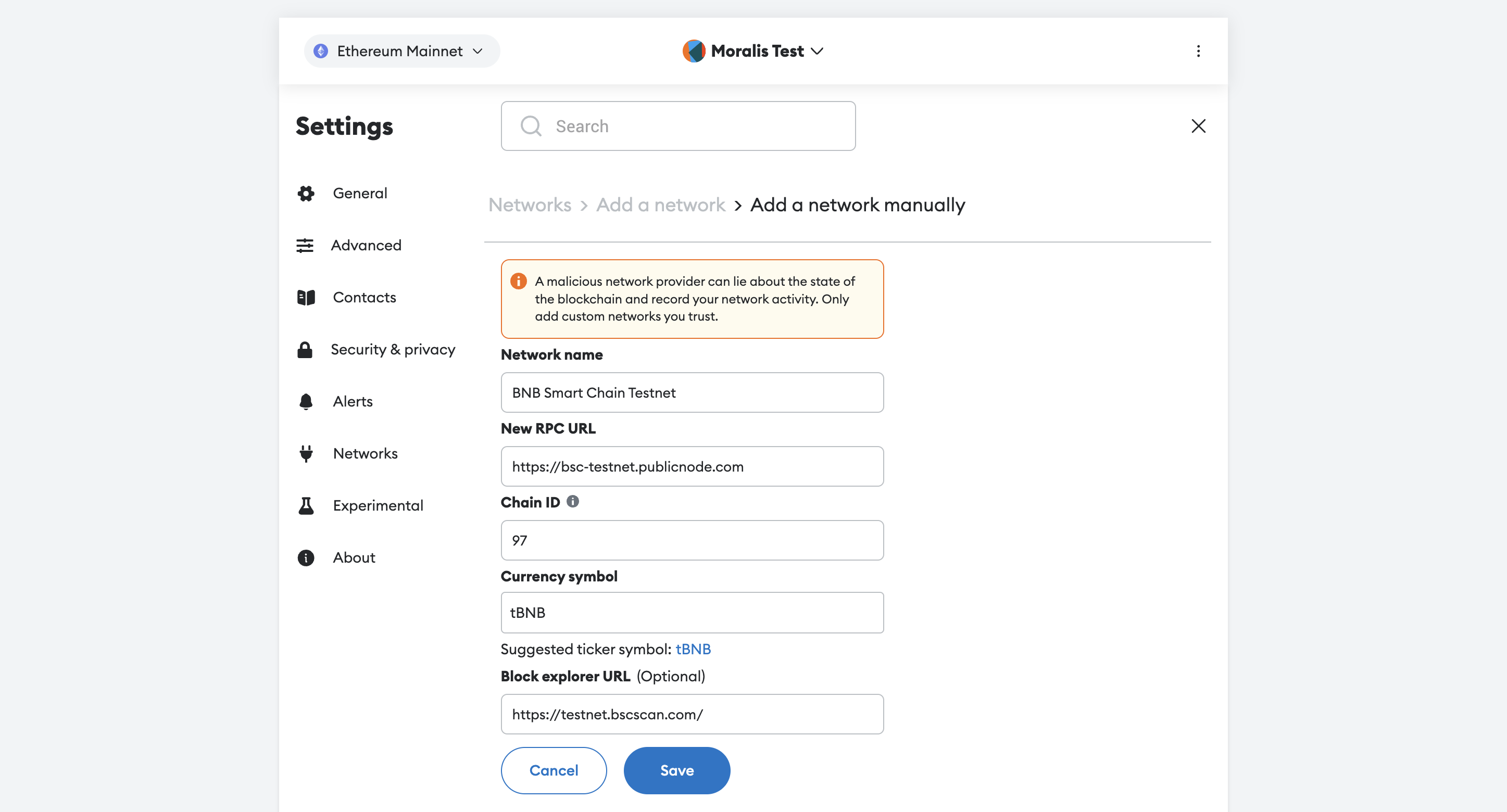
You’ll discover the data you want beneath:
After hitting “Save”, the community may have been added to your pockets, permitting you to modify to the testnet:
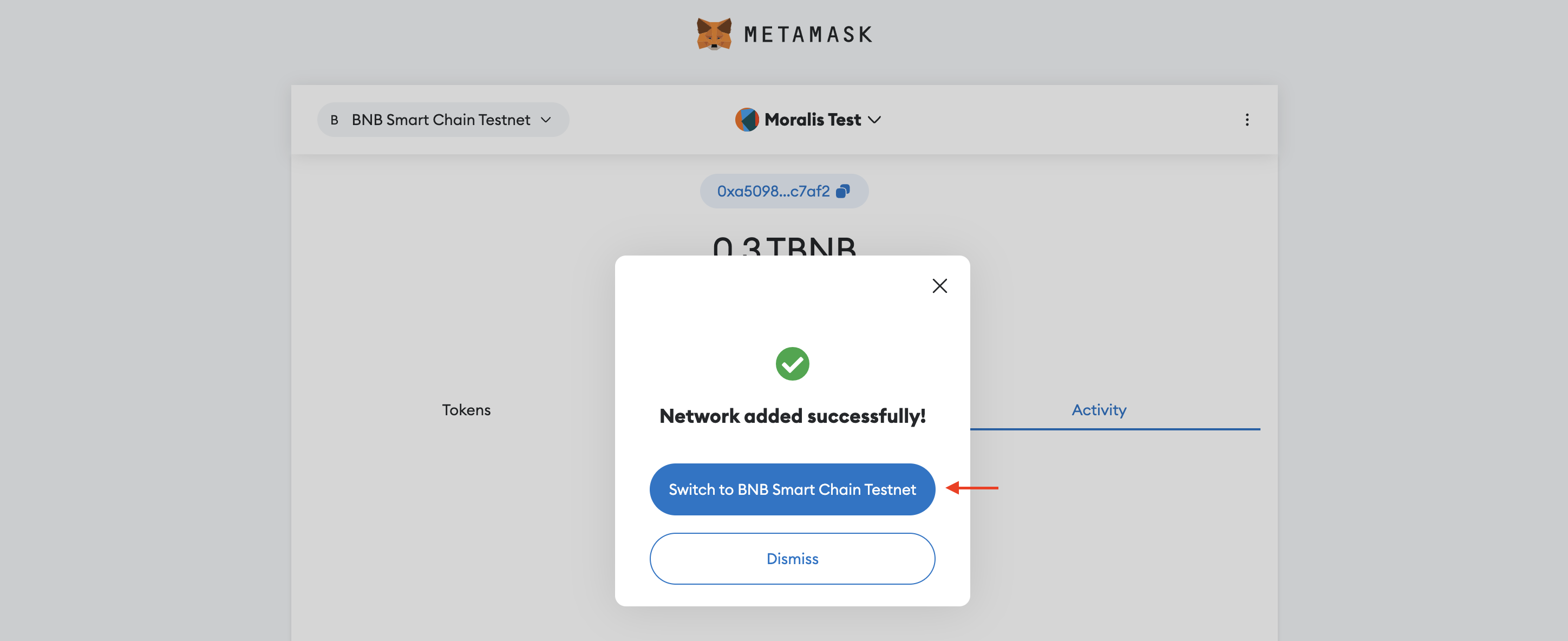
That’s it; you could have now efficiently added the BSC testnet to your MetaMask pockets:
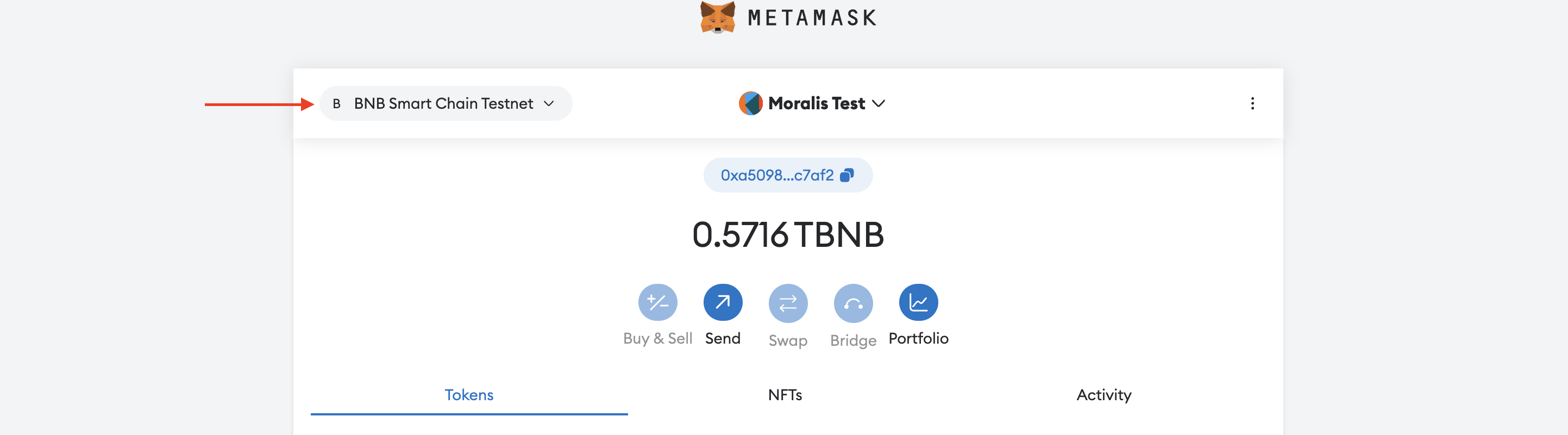
Step 2: Get BSC Testnet Tokens
To get BSC testnet tokens, you want a dependable BNB faucet, and the simplest technique to discover one is to make use of Moralis. Merely go to Moralis’ crypto taps web page, scroll down, and click on ”Attempt Now” for the BSC different:
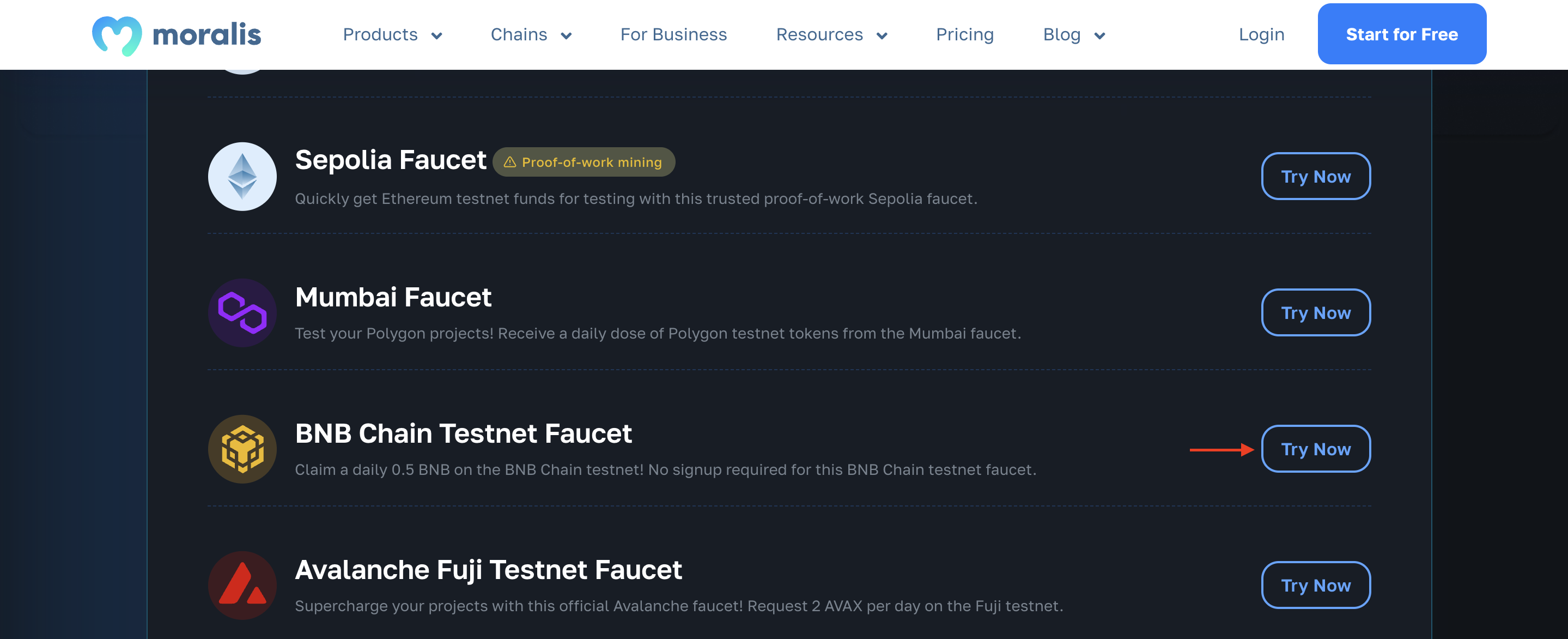
Clicking the button above takes you to the next web page, the place you simply want to stick your MetaMask tackle and hit the ”Ship 0.3 BNB” button:
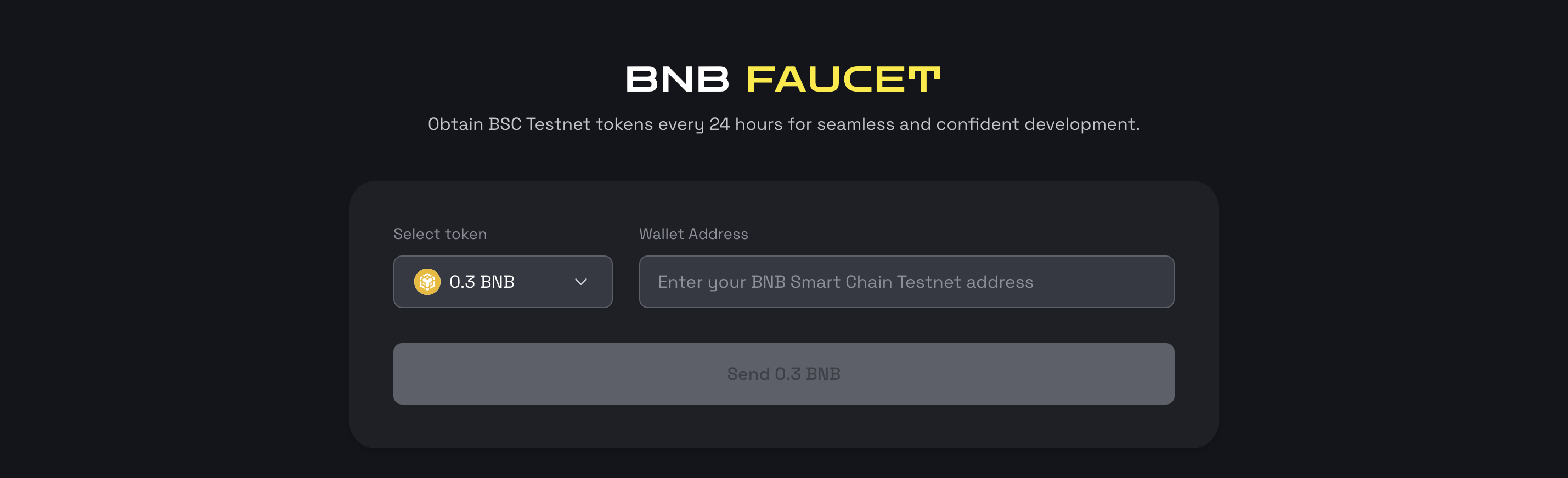
As soon as the transaction is finalized, it is best to now end up with an extra 0.3 BNB testnet tokens added to your MetaMask pockets:
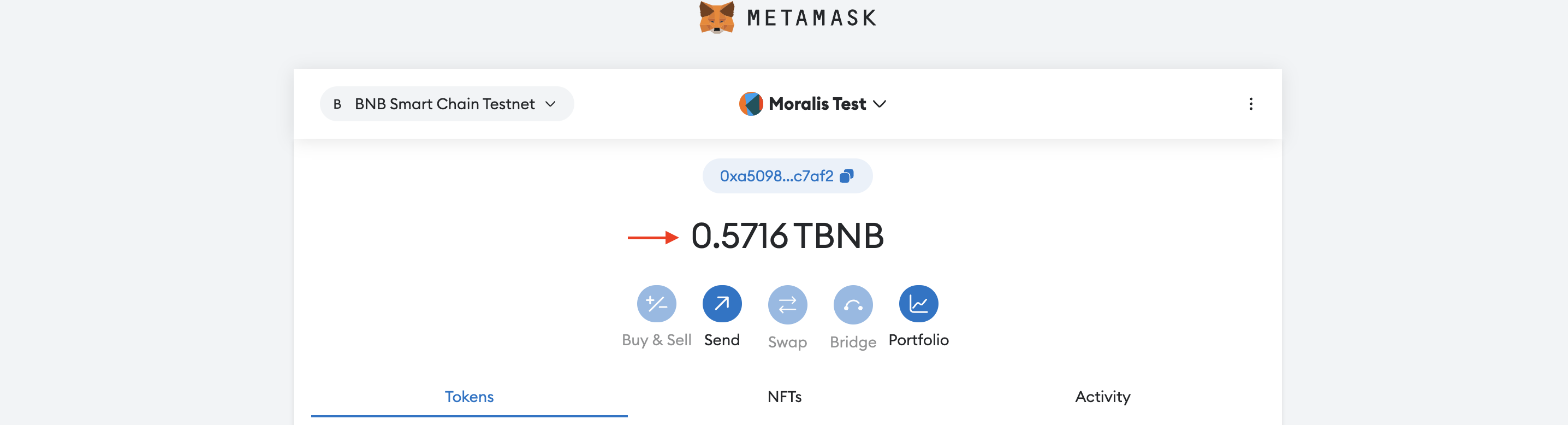
From right here, now you can use these newly acquired tokens to pay for transactions on the BSC testnet!
Step 3: Create Your BSC Token Contract with Remix
Since BSC is EVM-compatible, we will use Solidity and the ERC-20 token customary to create BEP-20 tokens. This additional means we will leverage an ERC-20 good contract template from OpenZeppelin to make this course of as seamless as doable.
With that mentioned, let’s begin by launching the Remix IDE and establishing a brand new workspace:
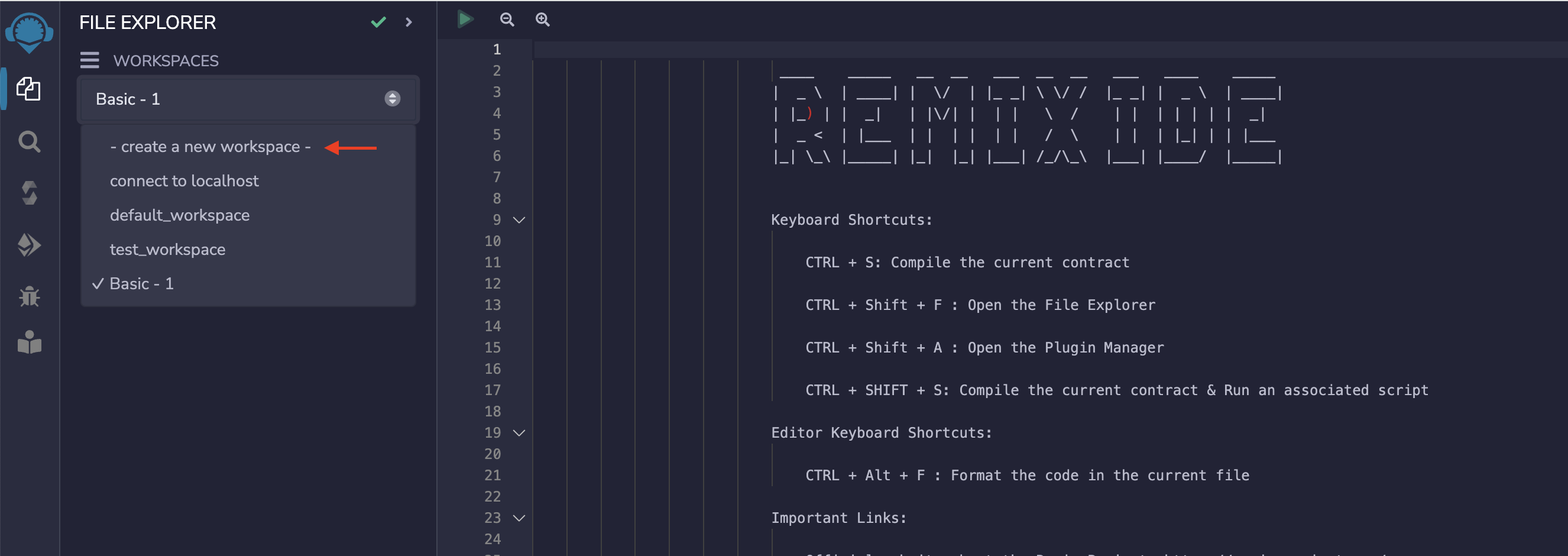
You’ll be able to then create a brand new good contract known as one thing like ”BEP20.sol” in your ”contracts” folder:
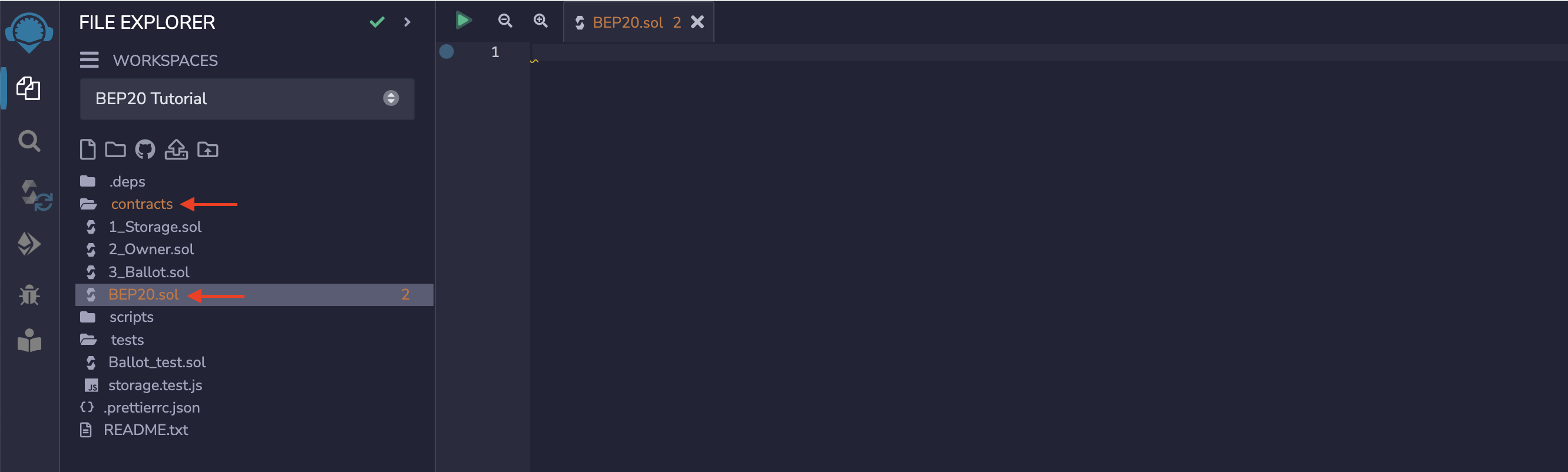
Subsequent, add the next code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC20/ERC20.sol”;
contract BEP20 is ERC20 {
constructor(uint256 initialSupply) ERC20(“BEP20Test”, “BPT”) {
_mint(msg.sender, initialSupply);
}
}
From right here, let’s now break down the code line by line, ranging from the highest!
We start by specifying the SPDX license:
// SPDX-License-Identifier: MIT
Subsequent, we import the ERC-20 bundle from OpenZeppelin:
import “@openzeppelin/contracts/token/ERC20/ERC20.sol”;
From there, we outline a brand new contract named BEP20, the place we additionally specify that it ought to use the ERC-20 bundle we imported above:
contract BEP20 is ERC20 {
//…
}
We then create a constructor, which is named when the good contract is deployed. This constructor takes an initialSupply parameter as an argument, which will probably be used to specify the preliminary provide of our token.
On the identical line, we moreover name the ERC20() perform we imported from OpenZeppelin. This perform takes two parameters as an argument: BEP20Test and BPT. The primary parameter is the identify of the token, and the second parameter is its ticker:
constructor(uint256 initialSupply) ERC20(“BEP20Test”, “BPT”) {
//…
}
Lastly, we name the _mint() perform, which is used to create the token. This perform takes two parameters: msg.sender and initialSupply. msg.sender specifies that the tokens will probably be despatched to the tackle deploying the contract, and initialSupply units the variety of tokens that will probably be deployed:
_mint(msg.sender, initialSupply);
Step 4: Deploy the Contract
At this level, it’s now time to deploy the good contract to the BSC testnet, and to take action, we initially must compile it. As such, go to the ”Solidity compiler” tab, choose your contract, and hit the ”Compile…” button:
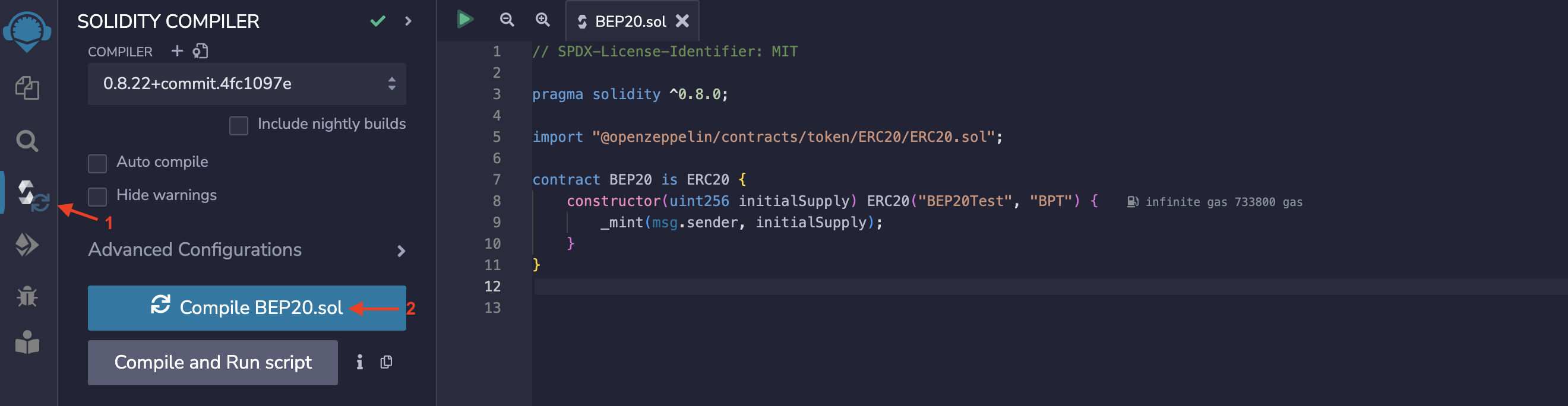
Subsequent, navigate to the ”Deploy & run transactions” tab and choose ”Injected Supplier – …” because the surroundings:
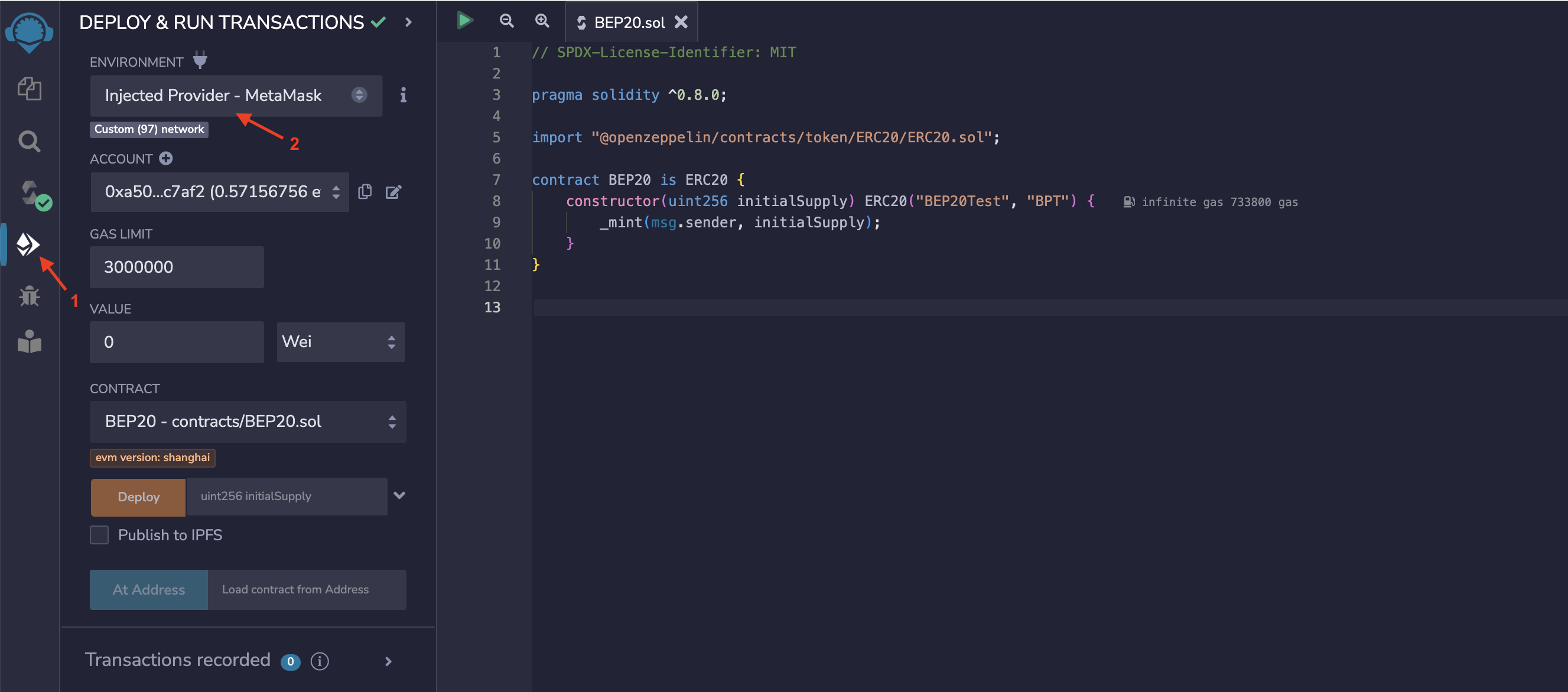
From right here, make sure that you’re deploying the best contract:
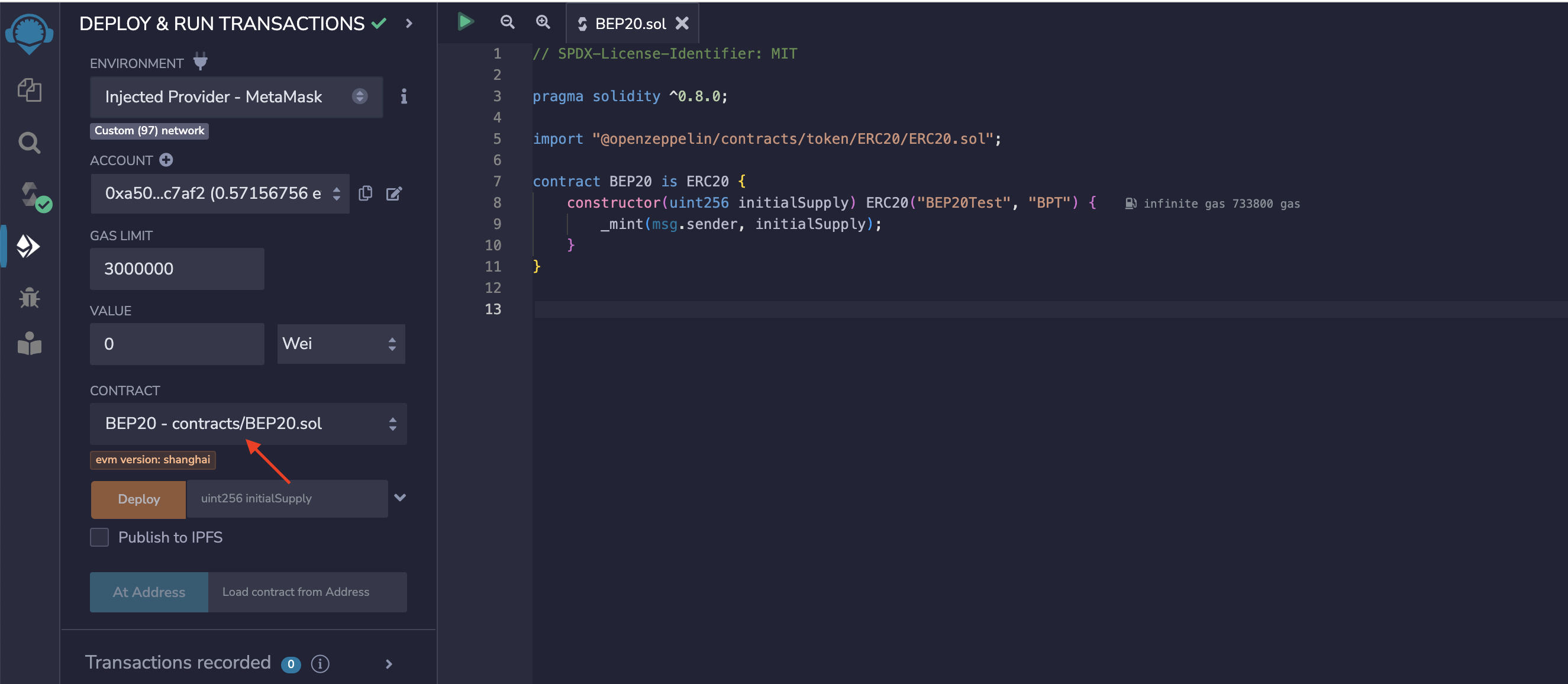
You then must specify the preliminary token provide that we cross to the contract’s constructor. In our case, we’ll be creating 100 tokens, and because it follows an 18-decimal format, we’ll cross 100000000000000000000 because the parameter:
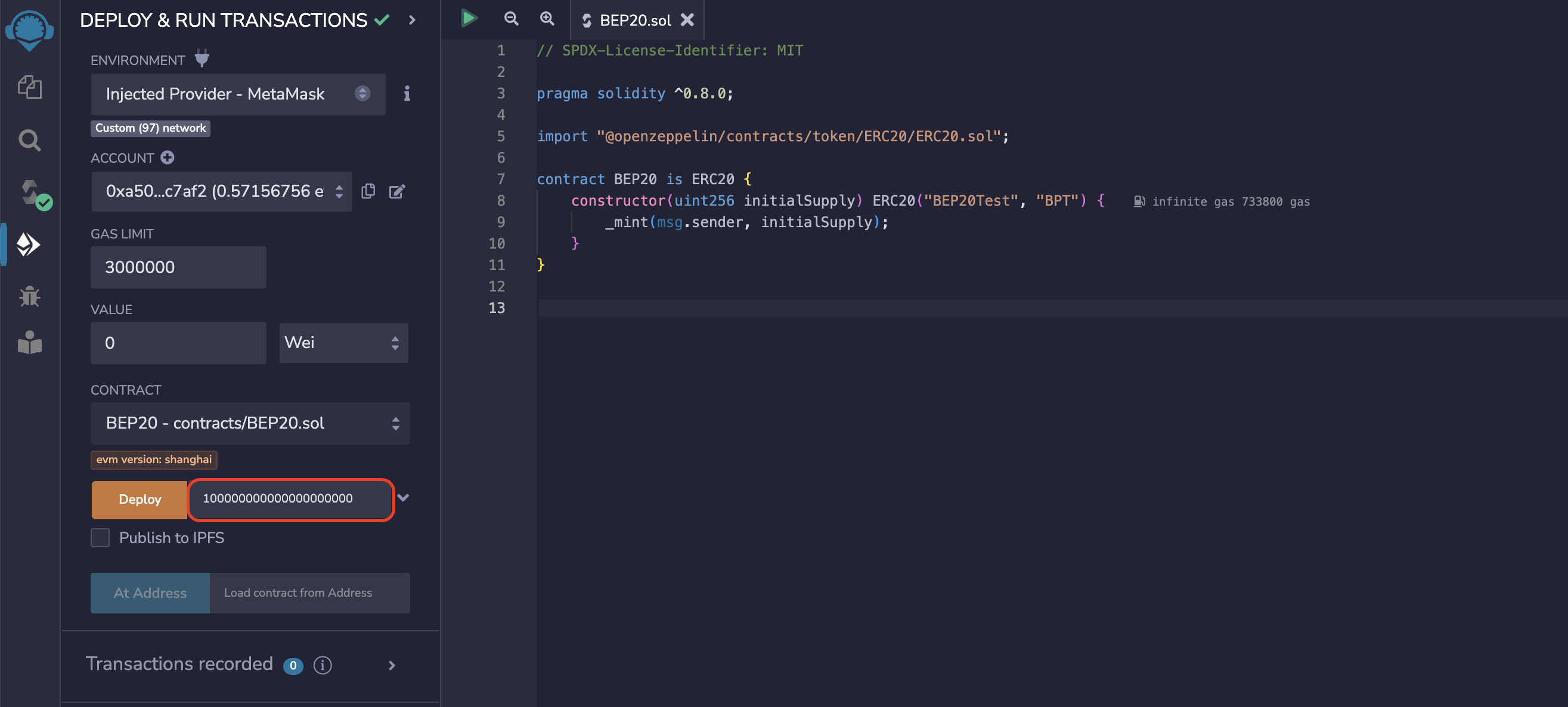
Lastly, all that is still is hitting the ”Deploy” button:
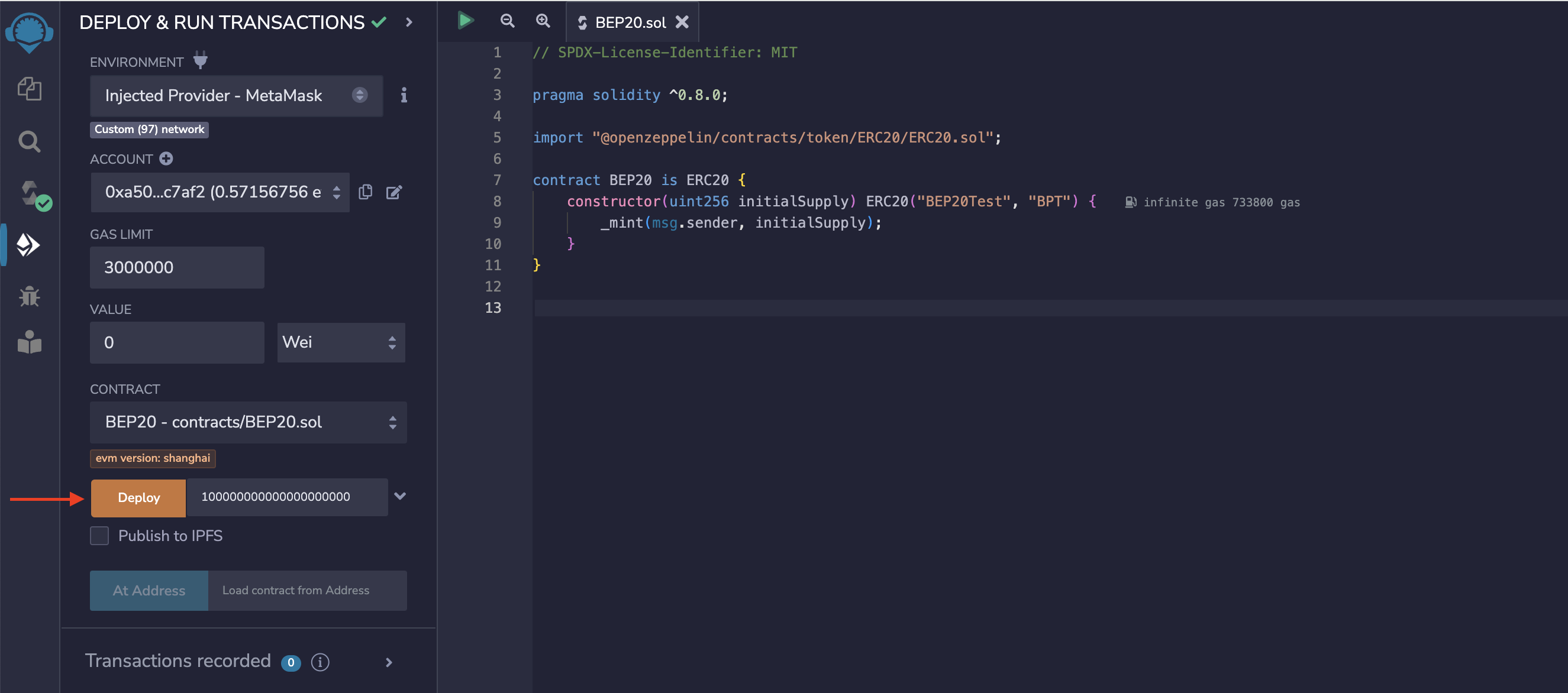
Clicking the ”Deploy” button prompts your MetaMask pockets, as you’ll must pay for the transaction utilizing the testnet tokens you beforehand acquired:
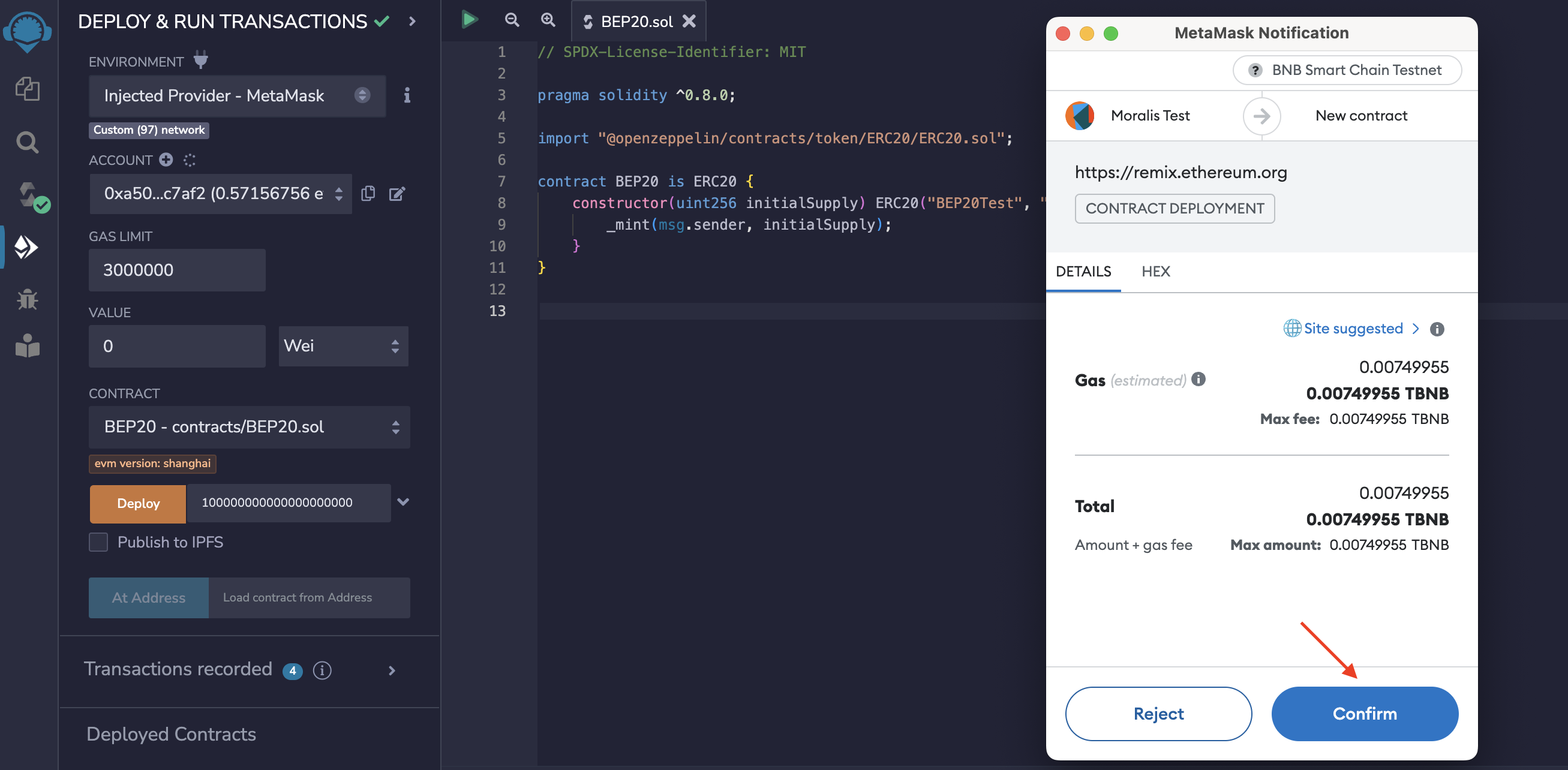
If all the things went based on plan, it is best to now see a hit message within the terminal:
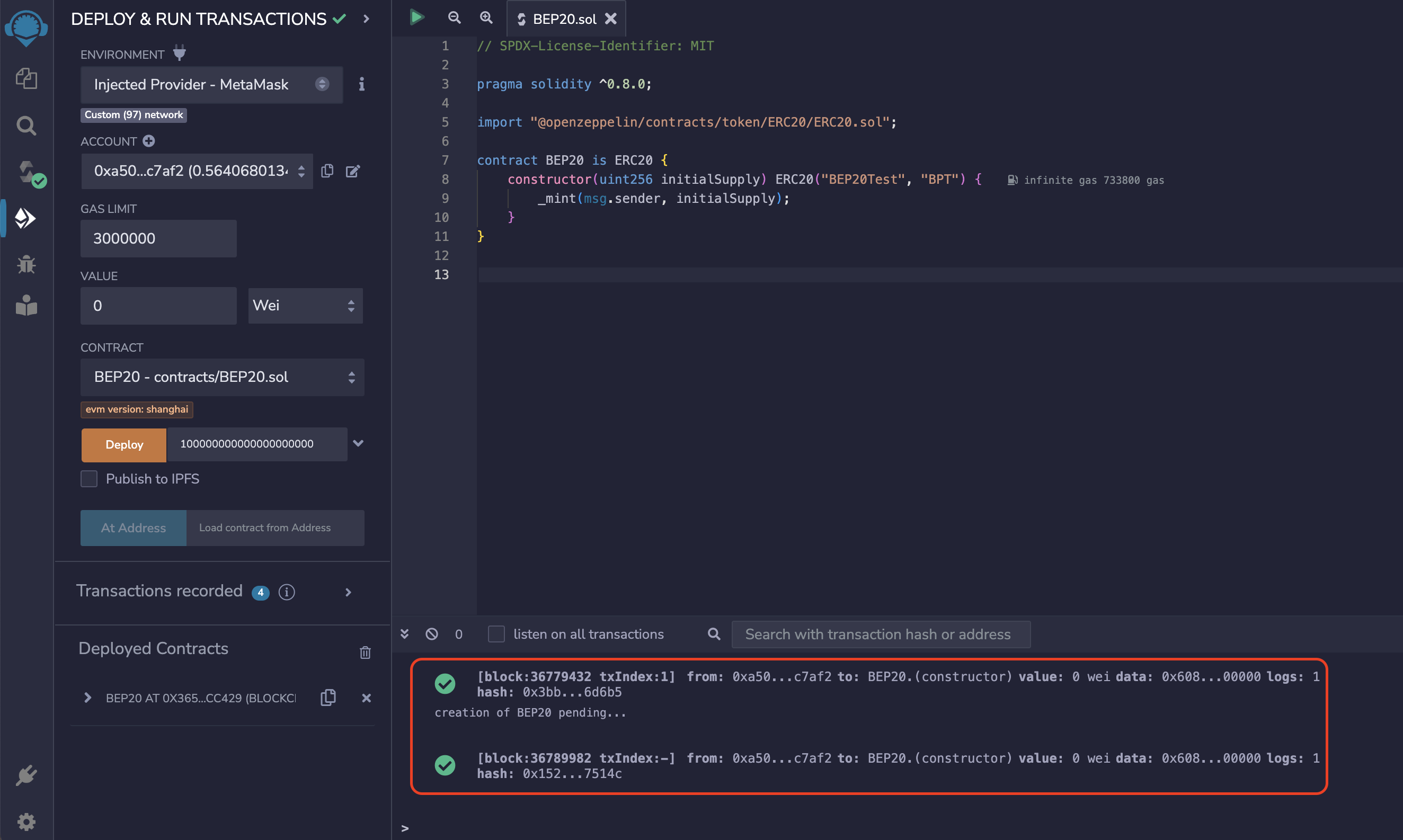
Step 5: Add the Token To MetaMask
So as to add the newly deployed token to your MetaMask pockets, you first want to repeat its contract tackle:
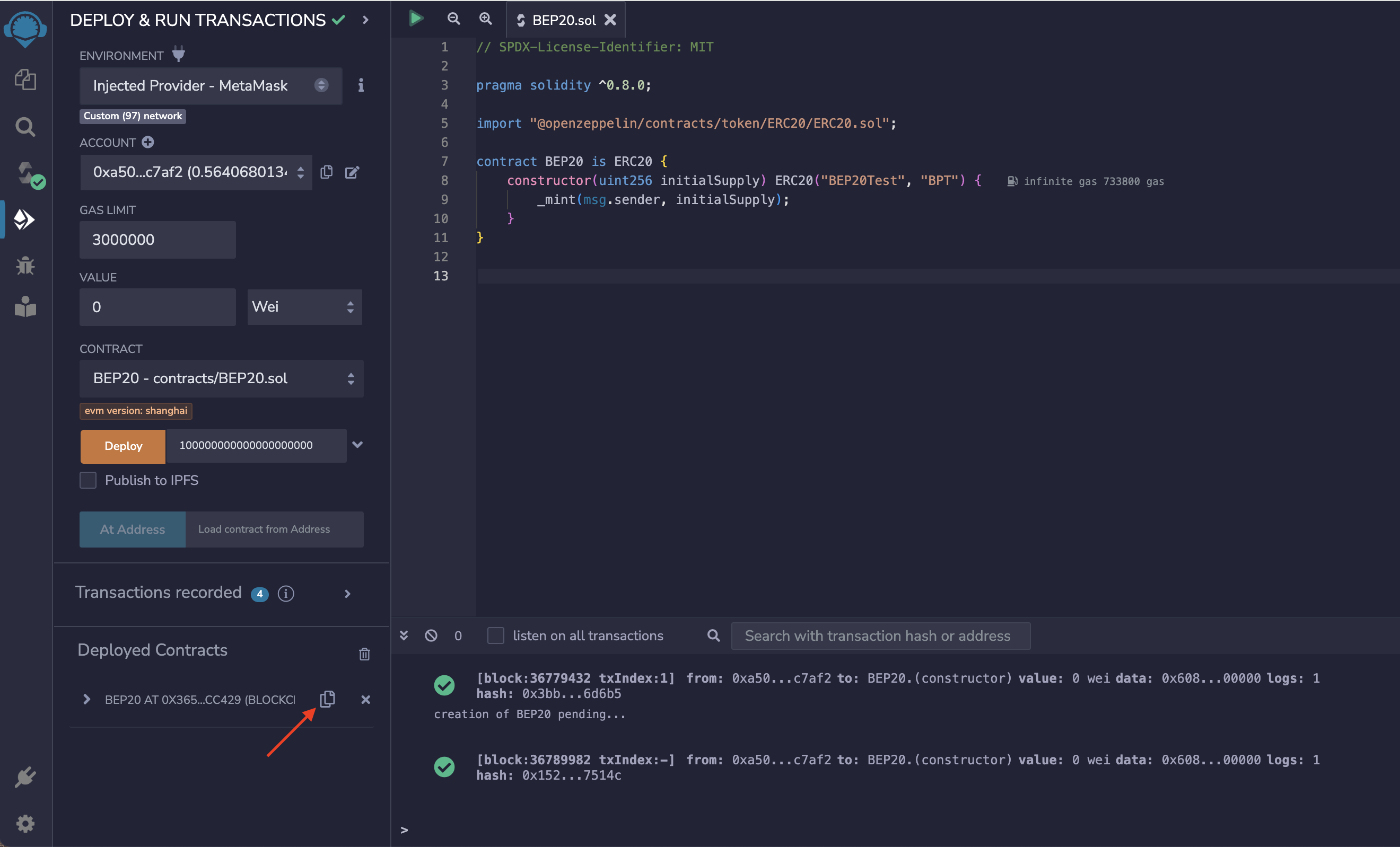
Subsequent, open your MetaMask pockets and click on the ”+ Import tokens” button:
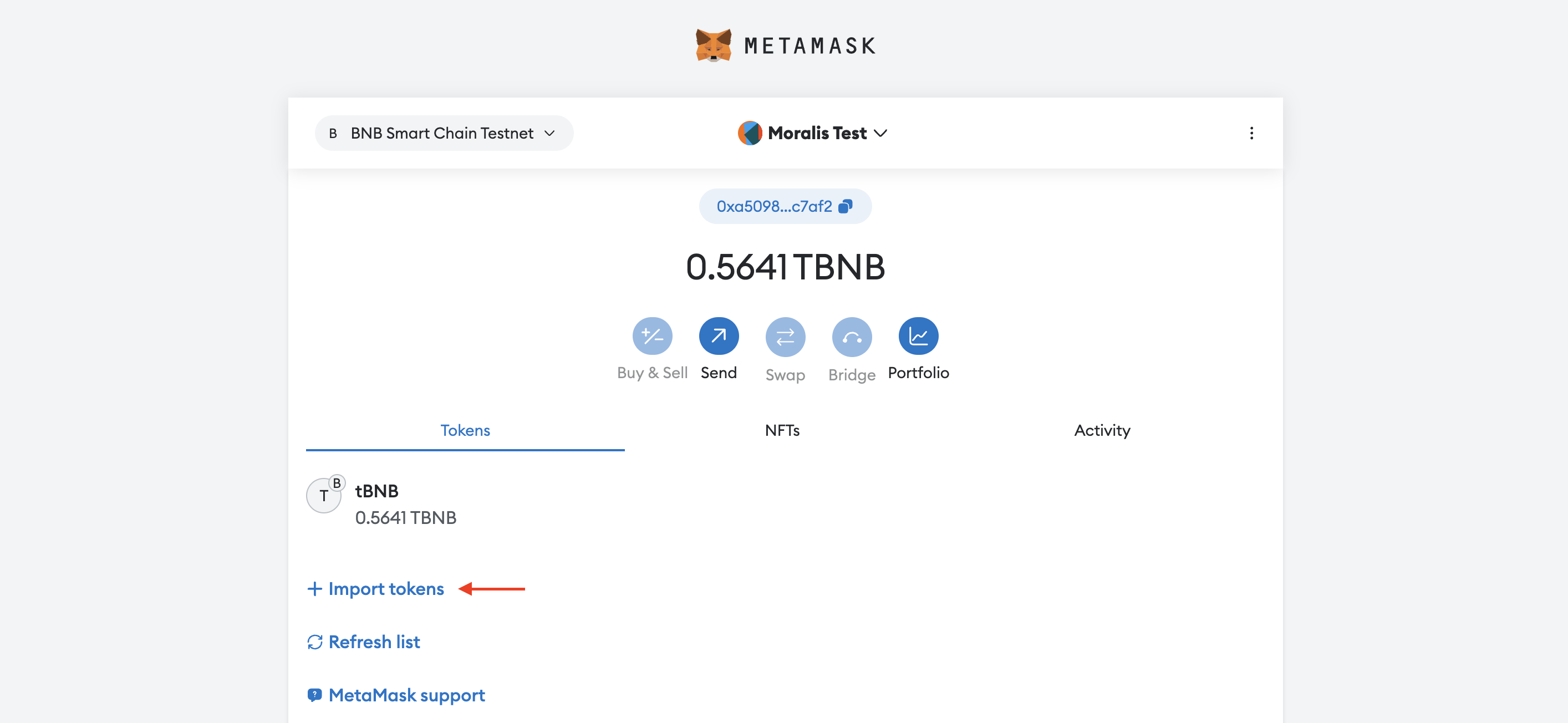
This can open a brand new window the place you merely want to stick the token tackle, and the remainder of the data ought to autonomously fill in:
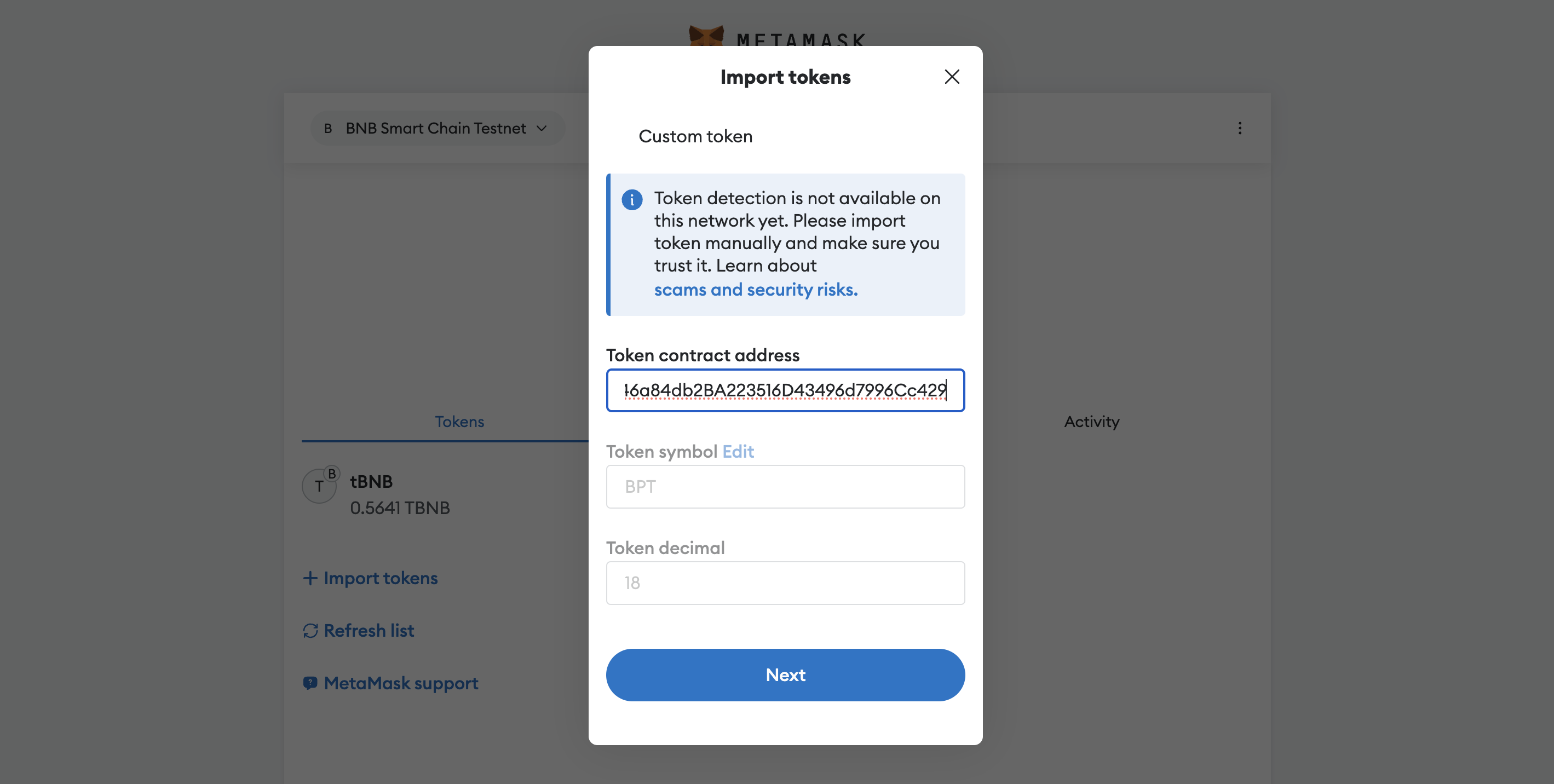
Lastly, click on ”Subsequent” adopted by ”Import”:
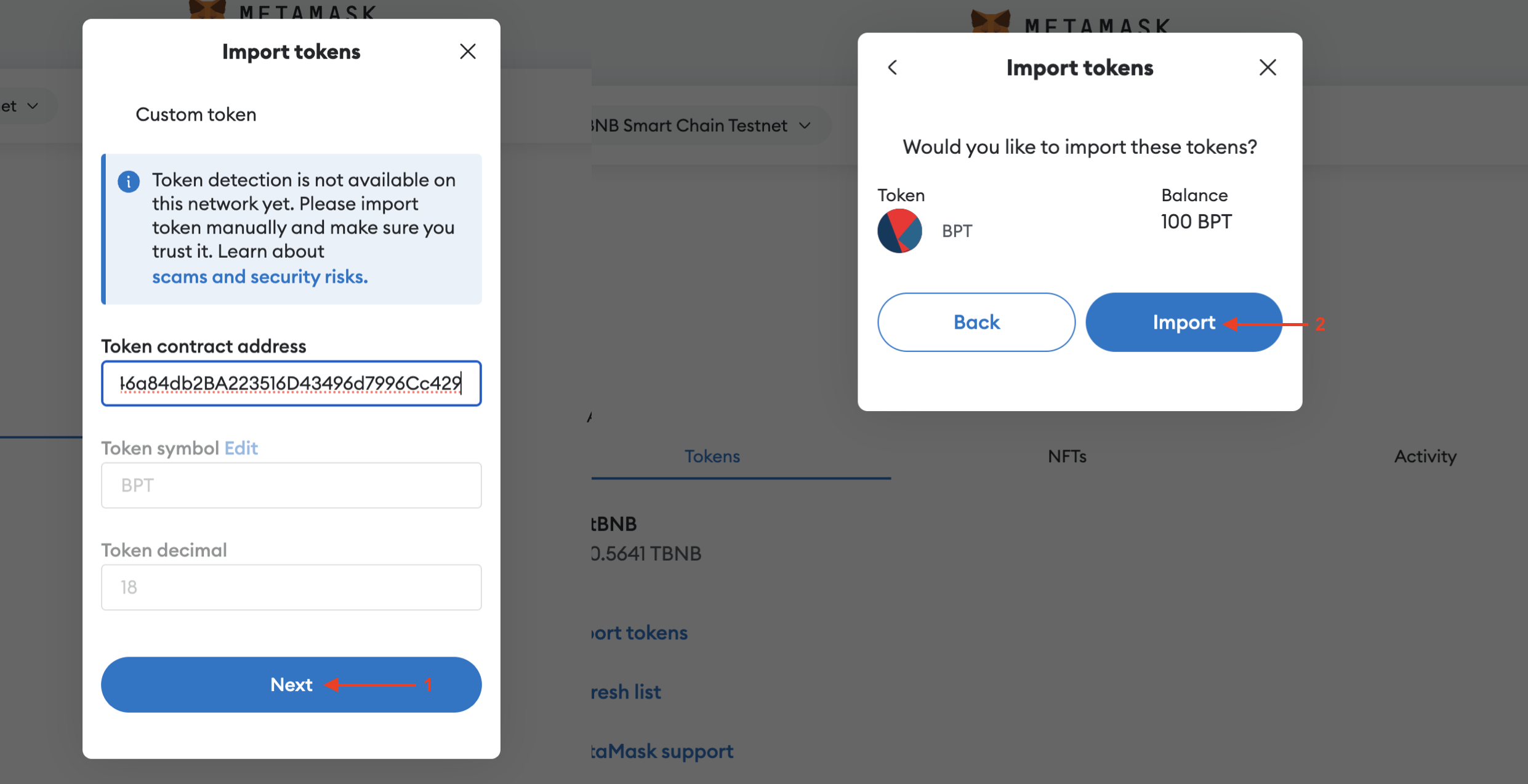
That’s it; it is best to now see your newly created BSC token in your MetaMask pockets:
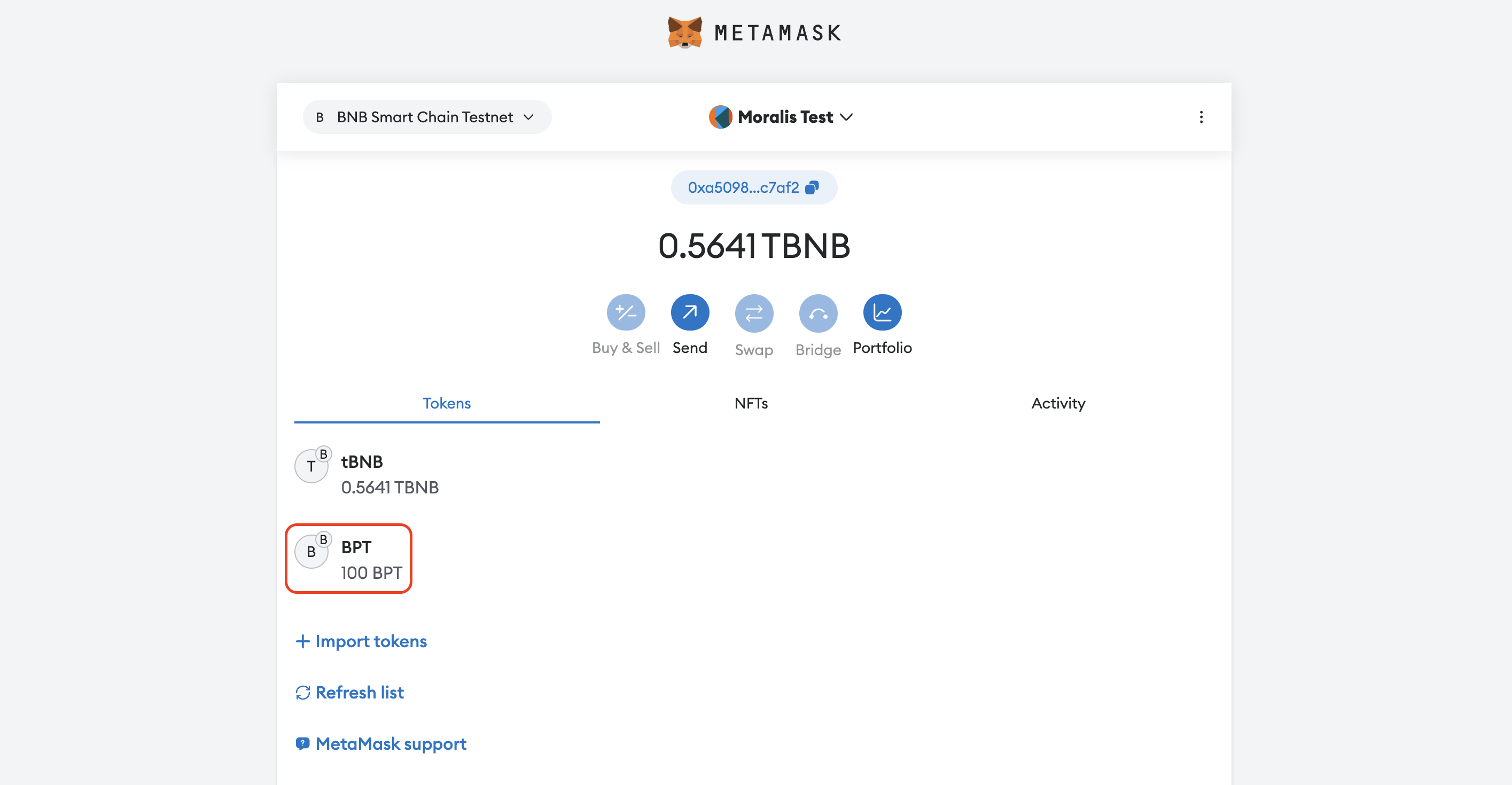
Congratulations! You now know how one can effortlessly create a token on the BSC testnet. From right here, you may comply with just about the identical steps to create a BSC token on the mainnet!
Introducing Moralis – The Business’s Main Web3 API Supplier
Moralis is the {industry}’s main Web3 API supplier, providing a large and dynamic vary of instruments you need to use to streamline your growth endeavors. In our suite of premier Web3 APIs, you’ll discover interfaces similar to our Pockets API, Token API, NFT API, Blockchain API, and plenty of others. Consequently, it doesn’t matter if you happen to construct a DEX, Web3 pockets, or some other platform; Moralis has a software for many use instances!
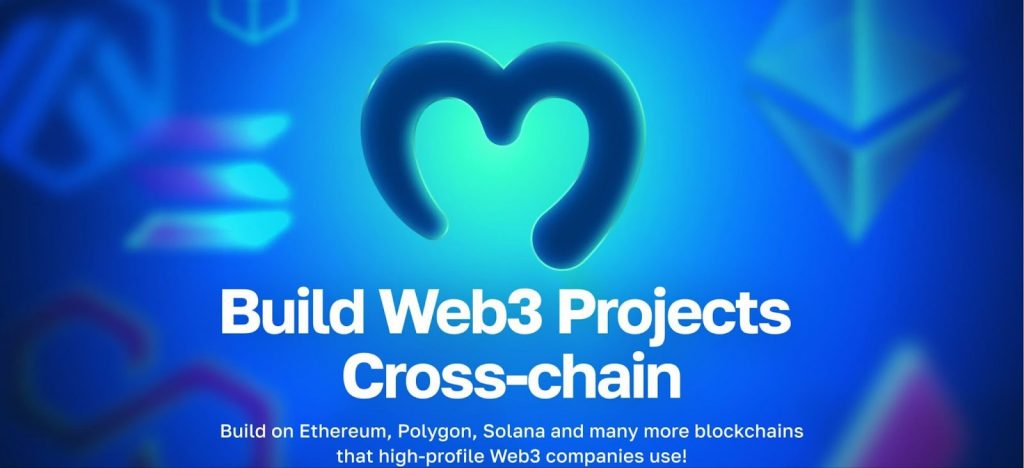
However why must you leverage Moralis when constructing dapps?
To reply the above query, let’s discover three advantages of Moralis!
High Efficiency: Moralis’ Web3 APIs persistently ship top-tier efficiency. It doesn’t matter whether or not you measure by reliability, pace, or some other metric; Moralis all the time blows the competitors out of the water. Knowledge Accessibility: With our various set of APIs, you get correct, real-time knowledge with only some traces of code. As such, when working with Morails, it has by no means been simpler to fetch and combine on-chain knowledge and Web3 performance into your tasks. Cross-Chain Compatability: Our Web3 APIs are chain-agnostic, supporting all the most important blockchains, together with networks like Ethereum, Polygon, Arbitrum, Solana, and, after all, BNB Good Chain, making our suite of instruments stand out as the last word BSC API.
To be taught extra about our industry-leading growth instruments, take a look at our Web3 API web page!
Additionally, keep in mind you can enroll with Moralis at no cost. So, take this chance, and you can begin constructing Web3 tasks quicker and extra effectively right now!
Abstract: Easy methods to Create a Token on BSC with Remix
In right now’s article, we kicked issues off by exploring the ins and outs of BSC tokens. In doing so, we discovered that they’re property constructed on BNB Good Chain adhering to the BEP-20 token customary.
From there, we then explored Remix and MetaMask. Remix is a distinguished IDE for writing, testing, debugging, compiling, and deploying EVM-compatible good contracts. In the meantime, MetaMask is a Web3 pockets for storing and managing digital property, together with interacting with dapps and good contracts.
Subsequent, we confirmed you how one can create a token on BSC with Remix in 5 easy steps:
Add the BSC Testnet to MetaMaskGet BSC Testnet TokensCreate Your BSC Token Contract with RemixDeploy Your ContractAdd the Token To MetaMask
As such, when you have adopted alongside this far, you now know how one can create a BSC token with Remix!
In case you favored this tutorial on how one can create a BSC token, take into account testing extra Moralis content material. For example, learn our contract ABI information or learn to record all of the cash in an ETH tackle.
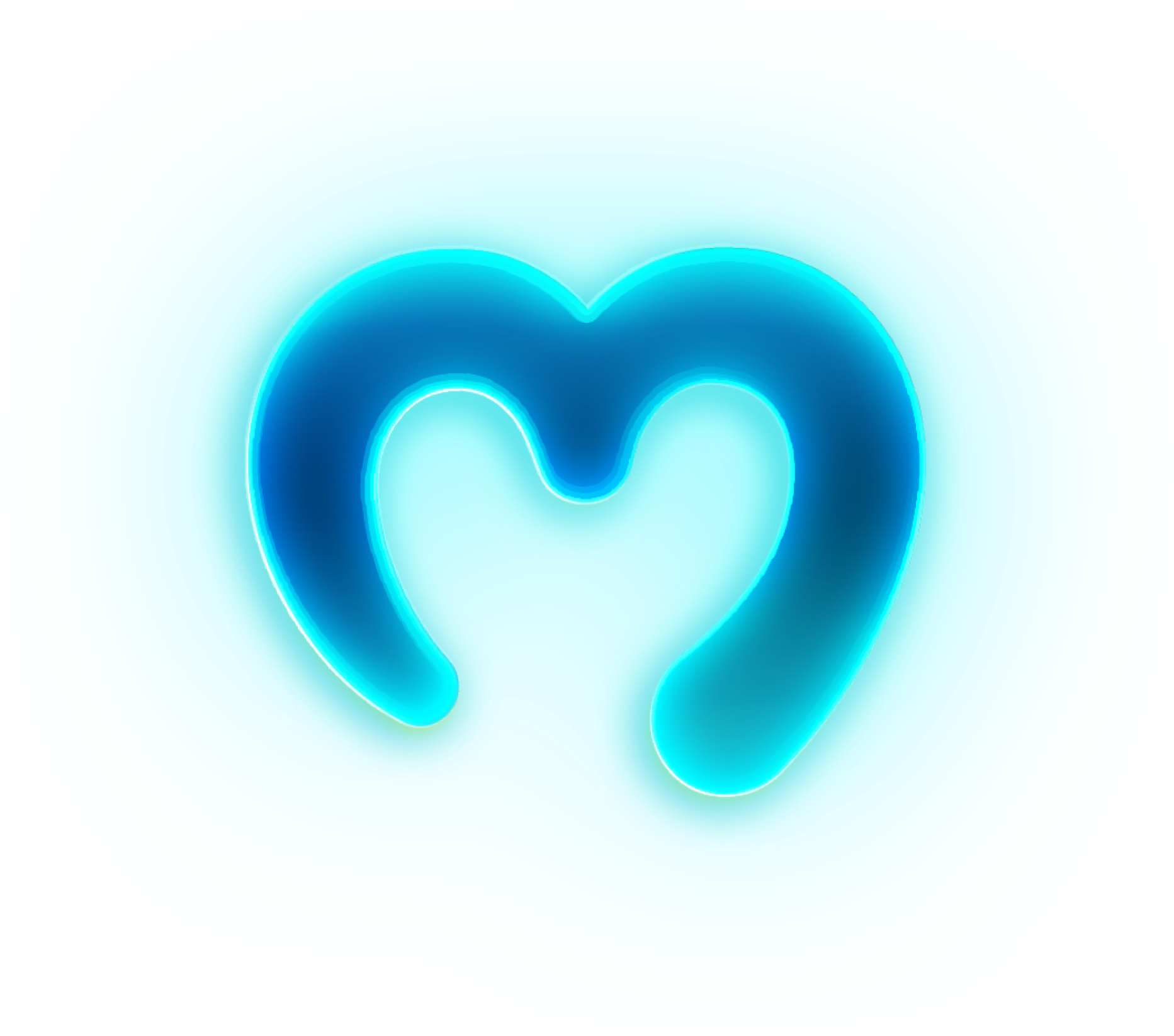
Moreover, for these excited to embark on their journey in Web3 growth, remember to enroll with Moralis. Signing up is free, and also you’ll acquire on the spot entry to all our premier Web3 APIs!